Python’s versatility and simplicity have made it a favorite among developers. At the heart of Python’s power lies its modular approach to code organization. Python modules are the building blocks that allow developers to break down complex programs into manageable and reusable components.
In this article, we embark on a journey to explore the world of Python modules. From creating and importing modules to harnessing the capabilities of the Python Standard Library and third-party modules, we’ll delve into every aspect of modular Python programming. Whether you’re a beginner looking to understand the fundamentals or an experienced developer seeking advanced insights, this article will equip you with the knowledge and skills to harness the true potential of Python modules in your projects. Let’s unlock the magic of modularity and elevate your Python programming to new heights.
What are Python Modules?
In the world of Python programming, modularity is a foundational principle that fosters efficient code organization and management. Python modules, the topic of this section, play a pivotal role in this regard. They are encapsulated units of code that facilitate the creation, organization, and reuse of code components. Here, we will provide a concise introduction to Python modules, shedding light on why they are indispensable for organizing and managing code effectively.
Python modules are files containing Python code, variables, functions, and classes. These files serve as self-contained units that can be imported and utilized in other Python scripts. The contents of a module can be accessed via importing, making them accessible for a wide range of applications.
The utility of Python modules stems from their ability to:
- Promote Code Reusability: Python modules allow you to encapsulate related code elements into separate files, making it easier to reuse the same code across multiple parts of a project or in entirely different projects.
- Enhance Code Organization: Modules help maintain code organization by breaking down complex programs into manageable, logical units. This makes code easier to understand, debug, and maintain.
- Reduce Code Duplication: Python modules minimize code duplication by centralizing code components in one place. If a change is required, it only needs to be made in one module, ensuring consistency across the project.
- Facilitate Collaborative Development: Modules are instrumental when collaborating on projects. Team members can work on separate modules simultaneously, leading to more efficient development processes.
In summary, Python modules are vital for achieving code modularity, fostering a well-structured, organized, and maintainable codebase. They form the foundation upon which complex Python applications and projects are built, allowing developers to harness the full potential of the language’s versatility and scalability. In the following sections, we will explore the practical aspects of creating, importing, and using Python modules to bring these benefits to life.
How can you create and import your own Python Modules?
Python’s modularity is one of its defining features, empowering developers to create structured and organized code by using modules. In this section, we’ll dive into the practical aspects of creating and importing modules in Python, equipping you with the skills to harness their power for code reusability and maintainability.
Creating a Python module is a straightforward process. Here’s how you can create your own module:
- Create a Python File: Start by creating a Python file with a
.py
extension. This file will contain the code you want to encapsulate in the module. - Define Functions, Variables, or Classes: Inside the Python file, define the functions, variables, or classes you want to include in the module. These elements should be related and serve a specific purpose within the module.
- Save the File: Save the file with a meaningful name that reflects the content and purpose of the module. The file name, excluding the
.py
extension, will be the module’s name when you import it.
Here’s an example of a simple Python module named “my_module.py”:
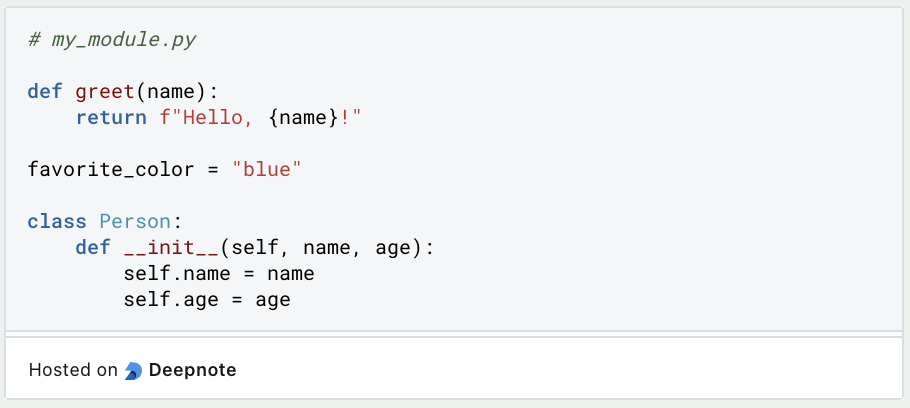
Once you’ve created a Python module, you can import and use it in other Python scripts. Python provides several ways to import modules:
- Import the Entire Module: You can import the entire module using the
import
keyword, followed by the module name (without the.py
extension). For example:

- Import Specific Elements: If you want to import specific functions, variables, or classes from a module, you can use the
from
keyword. For example:

- Using Aliases: You can assign aliases to Python module names or imported elements to make your code more concise and readable. For example:
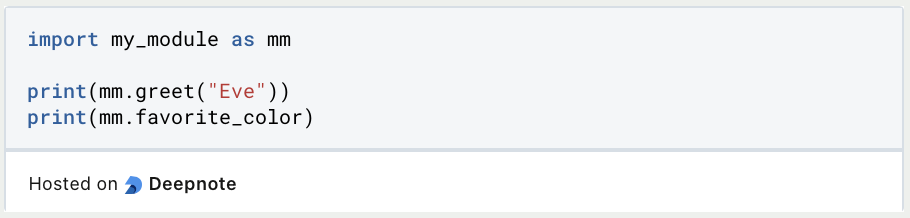
By creating and importing modules in Python, you establish a framework for organizing and managing your code. This promotes code reusability, enhances code organization, and reduces code duplication, making your projects more efficient and maintainable. In the following sections, we’ll explore more advanced techniques and best practices for working with modules in Python.
What are Standard Library Modules?
One of Python’s most remarkable features is its extensive Python Standard Library, a treasure trove of modules that provide a wide range of built-in functionality for a multitude of tasks. In this section, we’ll explore some key Python Standard Library modules, each designed to simplify and expedite common programming challenges.
- math – Module
- The
math
module is a powerhouse of mathematical functions, offering tools for operations like trigonometry, logarithms, exponentiation, and more. It’s invaluable for tasks that involve complex mathematical computations.
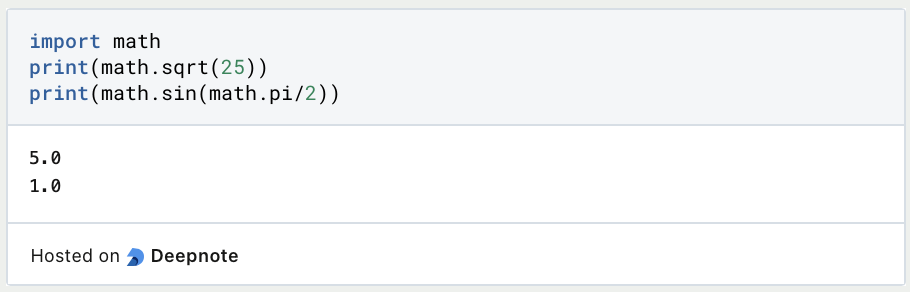
2. datetime – Module
- The
datetime
module is essential for working with dates and times. It enables the creation, manipulation, and formatting of date and time objects, making it indispensable in applications requiring time-related functionality.
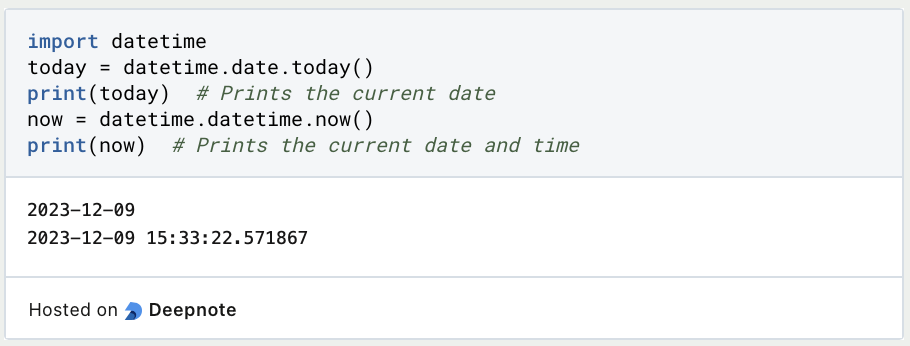
3. os – Module
- The
os
module provides a bridge between Python and the operating system, allowing you to interact with files, directories, and system functions. It’s crucial for tasks such as file manipulation, directory management, and system-specific operations.
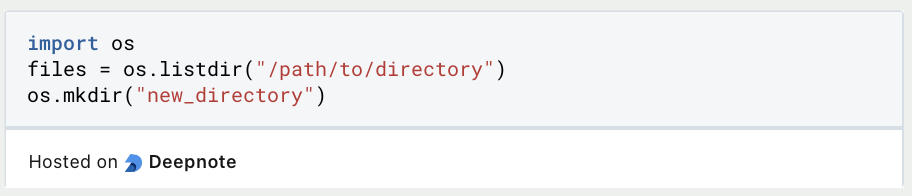
4. random – Module
- The
random
module serves as a versatile toolbox for generating random numbers, selecting random elements from sequences, and simulating random events. It’s invaluable for tasks involving randomness or probability.
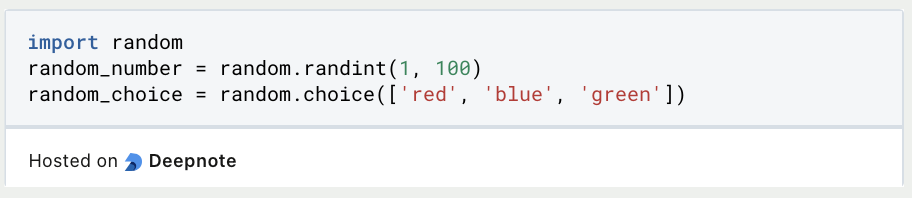
5. json – Module
- The
json
module is instrumental for working with JSON data, including parsing JSON strings and encoding Python objects into JSON format. It’s a cornerstone in applications that communicate with web services or handle configuration files.
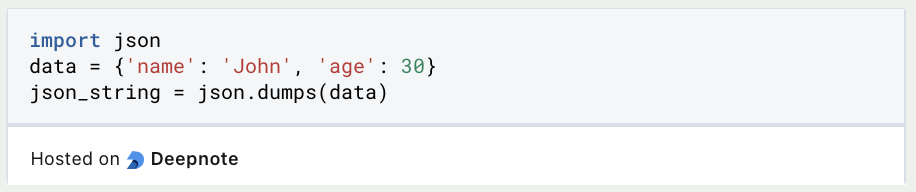
6. csv – Module
- The
csv
module simplifies the reading and writing of Comma-Separated Values (CSV) files, a common format for tabular data. It’s invaluable for data analysis, processing spreadsheets, and data exchange.
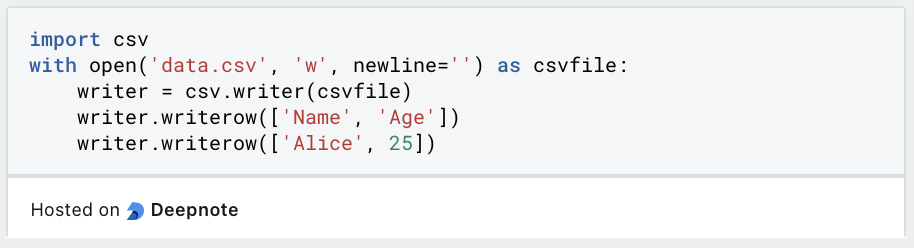
These are just a few examples of the myriad modules available in the Python Standard Library. The wealth of built-in functionality provided by these modules simplifies and expedites development, enabling you to focus on solving complex problems without reinventing the wheel. Whether you’re working with mathematics, dates, file systems, randomness, or data formats, the Python Standard Library is a valuable resource to explore.
What are Third-Party Python Modules?
While Python’s Standard Library provides a rich set of modules, it’s the third-party modules that truly expand the language’s potential. Third-party modules, created and maintained by the Python community, offer an extensive and diverse range of functionalities, addressing specialized needs, and extending Python’s capabilities. In this section, we’ll delve into the significance and use of third-party modules in Python programming.
The Role of Third-Party Modules
Python’s success as a versatile programming language is largely due to its vibrant community of developers who create and share third-party modules. These modules fill the gaps and provide solutions for specific use cases, enabling Python to adapt to a wide array of domains, from web development and data science to game development and machine learning.
The Python Package Index (PyPI)
The Python Package Index, commonly referred to as PyPI, is the central repository for third-party Python packages and modules. It hosts thousands of packages, each addressing various needs and requirements. Installing third-party modules from PyPI is made simple with Python’s package manager, pip
.
Installing Third-Party Modules
To install a third-party Python module, you can use pip
with the package name. For example, to install the popular NumPy library used in data science:

Common Third-Party Modules
There is a plethora of third-party modules available, catering to different domains and needs. Some notable examples include:
- NumPy: A fundamental library for numerical computing, offering support for large, multi-dimensional arrays and matrices.
- pandas: A powerful library for data manipulation and analysis, simplifying tasks like data cleaning, filtering, and aggregation.
- Django and Flask: Web frameworks that expedite web application development, handling routing, templating, and database interaction.
- Matplotlib and Seaborn: Data visualization libraries used for creating various types of charts and plots.
- TensorFlow and PyTorch: Deep learning frameworks popular in machine learning and artificial intelligence.
Choosing Third-Party Modules:
When selecting third-party modules, consider factors like community support, documentation, and compatibility with your project. It’s also important to verify that the module aligns with your project’s licensing and security requirements.
Best Practices for Managing Third-Party Modules
To ensure the smooth integration of third-party Python modules into your projects, follow best practices such as setting up virtual environments, creating clear dependencies with requirements.txt
, and keeping your modules updated.
Contributing to the Python Community
If you’re an experienced Python developer, consider contributing to the Python community by creating and maintaining your own third-party modules. Sharing your knowledge and solutions can benefit developers worldwide.
Third-party modules not only enhance the capabilities of Python but also encourage collaboration, innovation, and a dynamic ecosystem within the Python community. They empower developers to achieve remarkable feats in various fields and foster an environment of continuous learning and growth. As you explore the vast world of third-party Python modules, you’ll unlock the potential to tackle diverse and complex challenges with ease.
What is the Module Search Path?
The module search path, also known as sys.path
, is a fundamental aspect of Python’s module import mechanism. It plays a pivotal role in determining where Python looks for modules when the import
statement is used. Understanding the module search path is vital for efficient management and organization of modules within your Python projects.
At its core, the sys.path
list is a collection of directories that Python searches in a specific order to locate the modules you want to import. The order of directories in this list is crucial, as it defines the search priority. Here are the key aspects to comprehend regarding the module search path:
Python’s sys.path
list encompasses various default locations that the interpreter checks when importing modules. These include the directory where the current script is located, directories within the Python standard library, and site-specific paths that can be customized to suit your needs. The hierarchical order of these locations is significant, as Python first searches for modules in the current script’s directory and then proceeds to other predefined locations.
Modifying the sys.path
list is a useful technique when working with custom module directories. You can append directories to the list using the sys.path.append()
method or by directly manipulating the list. This enables Python to include additional directories in its search for modules. This can be beneficial when your modules are stored in non-standard locations, such as project-specific directories.
Furthermore, Python privileges the current script’s directory in the module search path. If a module with the same name as a Python standard library module exists in the current directory, it takes precedence. This can lead to unintended naming conflicts, emphasizing the importance of selecting module names that do not clash with standard library modules.
Virtual environments, created with tools like venv
or virtualenv
, offer a solution for managing project-specific dependencies and module search paths. Each virtual environment maintains its isolated sys.path
, ensuring that the required modules are available without interfering with the system-wide Python installation.
The PYTHONPATH
environment variable serves as another method for modifying the module search path at runtime. By setting the PYTHONPATH
variable, you can specify additional directories to include in sys.path
, further customizing the search path to accommodate your project’s needs.
In the context of packages, Python introduces an additional layer of complexity in the module search path. Each subdirectory of a package should contain a special __init__.py
file. This file can execute code and influence the package’s behavior, making it an integral part of package management.
Understanding the intricacies of the module search path is crucial for managing Python modules effectively, particularly in larger and more complex projects. It ensures that Python can locate the necessary modules, whether they belong to the standard library, third-party packages, or custom modules specific to your project. By strategically manipulating the module search path, you can enhance code organization, prevent naming conflicts, and improve the maintainability and portability of your Python projects.
What are Module Aliases?
Module aliases, a valuable feature in Python, allow developers to create shorthand references for modules when importing them. This practice not only enhances code readability but also mitigates potential naming conflicts, making it easier to work with modules that share similar names. In this section, we’ll explore the concept of module aliases, their practical use, and their significance in Python programming.
In Python, you can import modules using the import
statement, followed by the module name. For instance, to import the math
module:

While this method is straightforward, it can lead to verbose code when dealing with long module names or when multiple modules need to be imported. Module aliases offer a more concise and readable alternative. Here’s how to use module aliases:

In this example, we’ve introduced the alias m
for the math
module. Now, you can use the shorthand reference m
instead of the full module name. For example:

Module aliases are not limited to built-in Python modules; they can also be applied to third-party and custom modules. This flexibility allows you to create custom aliases that align with your project’s conventions and improve code readability. Consider the following example using a third-party module:

Python module aliases become particularly useful when you have modules with similar names or when there’s a risk of naming conflicts. For instance, if you’re working with multiple modules that share common names like utilities
or config
, using aliases helps you distinguish between them and avoid conflicts:

This way, you can identify which specific utilities
or config
module you’re referencing in your code.
In summary, Python module aliases are a practical and efficient way to streamline module imports. By assigning aliases, you can enhance code readability, reduce verbosity, and mitigate potential naming conflicts. Whether you’re working with built-in, third-party, or custom modules, module aliases are a valuable tool for managing and organizing your Python code.
What are special Python Module Attributes?
Python modules aren’t just containers for functions, variables, and classes; they also possess special module-level attributes that provide valuable information and functionality. In this section, we’ll delve into these special module attributes, exploring their significance and how they can be utilized in Python programming.
__name__
The __name__
attribute is a ubiquitous and versatile module attribute. When a module is executed directly (as the main program), its __name__
attribute is set to "__main__"
. This attribute is often used to create code that can be executed when the module is run as the main program but not when it’s imported as a module.

__file__
The __file__
attribute provides the full path to the module’s source file. It’s especially useful for locating the module’s source code, which can be beneficial in debugging or for situations where you need to manipulate files related to the module.

__doc__
The __doc__
attribute contains the module’s docstring, which is typically a string that describes the module’s purpose and functionality. Accessing this attribute can provide valuable information about the module’s intended use.

__all__
The __all__
attribute is a list that specifies which symbols (functions, variables, classes) should be considered as public symbols when the module is imported using the wildcard import statement from module import *
. It helps define the module’s public API.

__path__
The __path__
attribute is utilized in package modules. It contains a list of directory paths where Python searches for submodules within a package. This attribute can be useful for customizing the import behavior of package submodules.

These special module attributes serve distinct purposes in Python programming. While __name__
aids in creating versatile modules that can function as standalone programs or as importable components, __file__
facilitates file manipulation. The __doc__
attribute enhances code documentation and helps convey a module’s purpose to other developers. __all__
defines a module’s public interface, simplifying import operations, and __path__
offers control over the submodule search behavior within package modules.
Leveraging these special attributes allows developers to write more versatile and informative modules, making Python code more readable, maintainable, and adaptable. Understanding the role of these attributes in modules is essential for proficient Python programming.
Local vs. Global Scope
Python modules play a vital role in code organization, allowing for the encapsulation of code components into separate files. When working with modules, it’s essential to understand the concept of local and global scope, as it determines the accessibility of variables within a module and across modules. In this section, we’ll explore the distinctions between local and global scope in Python modules and how they impact variable management.
1. Local Scope
Local scope refers to the innermost level of variable visibility, which is limited to a specific block of code, such as a function or a class method. Variables defined within this scope are considered local variables. They are only accessible within the block where they are defined.
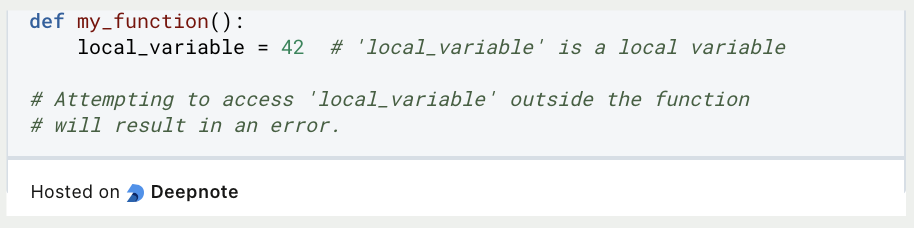
2. Global Scope
Global scope encompasses the broader context of a Python module or script. Variables defined at the module level, outside any function or class, are considered global variables. They can be accessed and modified from any part of the module.
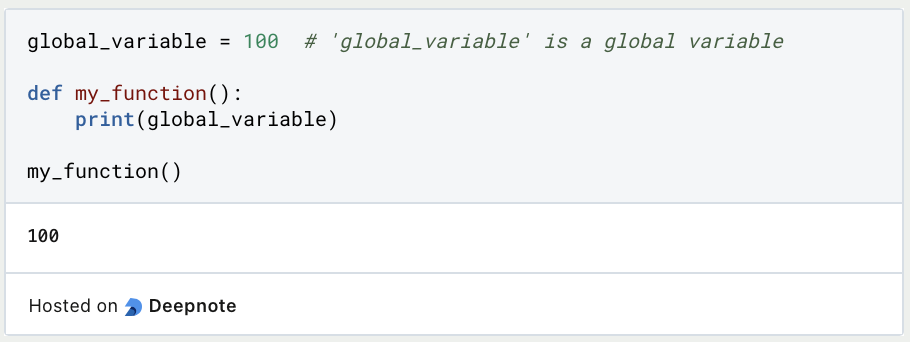
3. Accessing Variables
In Python, variables are resolved in a specific order known as the LEGB rule, which stands for Local, Enclosing (non-local), Global, and Built-in. When you reference a variable, Python first looks for it in the local scope. If not found, it moves up the scope hierarchy to find the variable in the nearest enclosing scope, then in the global scope, and finally in the built-in scope (containing Python’s built-in functions and variables).
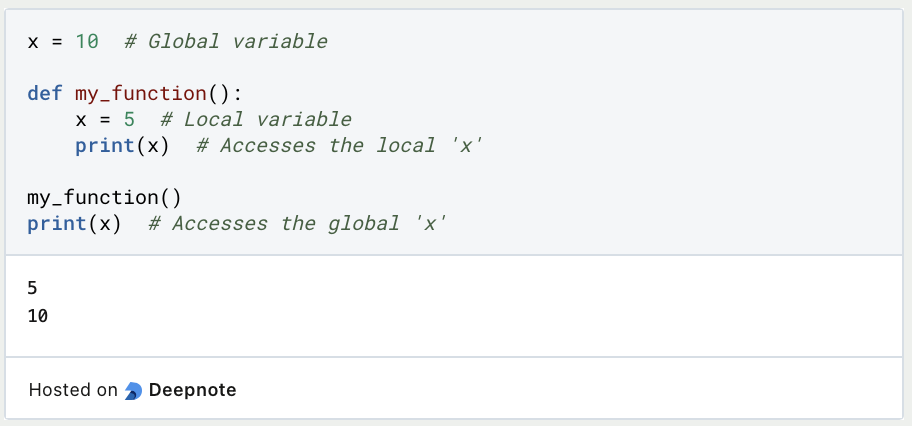
4. Modifying Variables
To modify a global variable from within a local scope, you need to use the global
keyword. This informs Python that you intend to modify the global variable rather than creating a new local variable with the same name.

5. Best Practices
It’s advisable to limit the use of global variables to essential cases, as excessive reliance on global scope can make code less readable and maintainable. Favor local variables within functions to encapsulate logic and reduce the risk of naming conflicts.
Understanding the interplay between local and global scope is crucial for effective variable management within Python modules. It enables you to create organized, modular, and maintainable code, whether you’re working with simple scripts or complex software projects. Carefully selecting the appropriate scope for variables ensures that your code behaves as expected and functions as part of a cohesive whole.
This is what you should take with you
- Python modules allow you to break down your code into manageable, organized units, making your projects more structured and maintainable.
- The
import
statement brings modules to life, allowing you to access their functions, variables, and classes effortlessly. - The Python Standard Library is a treasure trove of modules that provide a wide array of built-in functionality, from mathematics and date handling to file manipulation and data serialization.
- Don’t limit yourself to the standard library; explore the extensive world of third-party modules on the Python Package Index (PyPI). These modules cover diverse domains, from web development and data science to machine learning and game development.
- Understanding how Python searches for modules in the
sys.path
list is crucial. It helps you organize your code, manage dependencies, and prevent naming conflicts. - Module aliases are your allies for creating concise and readable code. They enhance code readability and help you avoid naming clashes when working with modules of similar names.
- Leverage special module attributes like
__name__
,__file__
, and__doc__
to provide context and control over your modules. These attributes enhance code documentation and execution flexibility. - Local and global scope are essential concepts in module development. Carefully manage variables within these scopes to maintain code clarity and prevent naming conflicts.
What is Jenkins?
Mastering Jenkins: Streamline DevOps with Powerful Automation. Learn CI/CD Concepts & Boost Software Delivery.
What are Conditional Statements in Python?
Learn how to use conditional statements in Python. Understand if-else, nested if, and elif statements for efficient programming.
What is XOR?
Explore XOR: The Exclusive OR operator's role in logic, encryption, math, AI, and technology.
How can you do Python Exception Handling?
Unlocking the Art of Python Exception Handling: Best Practices, Tips, and Key Differences Between Python 2 and Python 3.
What are Python Comparison Operators?
Master Python comparison operators for precise logic and decision-making in programming.
What are Python Inputs and Outputs?
Master Python Inputs and Outputs: Explore inputs, outputs, and file handling in Python programming efficiently.
Other Articles on the Topic of Python Modules
Here you can find the documentation on Python Modules of the official Python website.

Niklas Lang
I have been working as a machine learning engineer and software developer since 2020 and am passionate about the world of data, algorithms and software development. In addition to my work in the field, I teach at several German universities, including the IU International University of Applied Sciences and the Baden-Württemberg Cooperative State University, in the fields of data science, mathematics and business analytics.
My goal is to present complex topics such as statistics and machine learning in a way that makes them not only understandable, but also exciting and tangible. I combine practical experience from industry with sound theoretical foundations to prepare my students in the best possible way for the challenges of the data world.