Debugging is an essential part of the software development process. It is the process of finding and fixing errors or bugs in the code. Debugging can be a time-consuming and challenging task, but it is crucial for producing high-quality and reliable software. Python, being a popular programming language, provides various tools and techniques for debugging code.
In this article, we will discuss some of the most commonly used debugging techniques and tools in Python, and how they can be applied to identify and resolve issues in code.
What is Debugging?
Debugging is an essential process in software development that involves identifying and fixing errors in the code. It is a crucial skill for any developer, as even the most experienced programmers make mistakes. Debugging allows you to find and fix problems in your code, which helps improve the quality of your software. There are various debugging techniques and tools available in Python that make it easier to identify and resolve issues in your code. In this article, we will explore some of the most useful debugging techniques and tools in Python.
What are common Debugging issues?
Debugging is a crucial part of the software development process. Even experienced developers may encounter issues during the coding process, and debugging becomes an essential tool to find and fix problems. In Python, developers can come across many types of bugs, which can be frustrating to deal with. Here are some common debugging issues that developers face in Python.
- Syntax Errors: These are some of the most common issues that developers face when writing code. A syntax error occurs when the Python interpreter cannot parse the code because of incorrect syntax. The interpreter highlights the line where the error occurred, making it easy to fix.
- NameError: This occurs when a variable is used before it is defined. It is common in Python to forget to initialize a variable before using it, which can lead to a NameError.
- TypeErrors: These errors occur when you try to perform an operation on a data type that does not support that operation. For instance, trying to add a string to an integer can result in a TypeError.
- IndexErrors: These occur when you try to access an index that does not exist in a list. For example, if you have a list with three elements and you try to access the fourth element, you will get an IndexError.
- ValueErrors: These occur when you pass a value to a function that is not supported by that function. For instance, passing a string to a function that only takes integers will result in a ValueError.
- Logical Errors: These are more difficult to spot because the code will not throw an error. Instead, the output of the code will not be what you expect. Logical errors can be caused by incorrect assumptions or misunderstandings of the problem being solved.
Debugging can be time-consuming, but it is essential for ensuring that your code is working as expected. Knowing the common debugging issues in Python and how to solve them can make the process more manageable.
What are common tools for Debugging in Python?
Common tools used for debugging in Python include:
- print() statements: The easiest and most common way to debug code is to use print() statements to print out the values of variables at various points in the code. This allows you to see the values of variables and how they change as the code executes.
- Debuggers: Python has built-in debuggers such as pdb (Python Debugger) and ipdb (IPython Debugger) that allow you to step through code and examine the state of variables at each step. These debuggers can be run from the command line or integrated into an IDE.
- Logging: Python’s built-in logging module provides a more flexible and scalable way to log information about the execution of a program. Logging can help you understand the flow of control in your code and identify errors that occur during execution.
- Assertions: Assertions are statements in your code that check if a condition is true. If the condition is false, the program will raise an AssertionError. Assertions can be used to check if a function is being called with the correct arguments or if the output of a function is what you expect it to be.
- IDEs (Integrated Development Environments): IDEs such as PyCharm and Visual Studio Code offer built-in debugging tools that allow you to step through code, set breakpoints, and examine the state of variables at each step. These tools provide a more streamlined debugging experience and are especially useful for large projects with complex code.
- Third-party libraries: There are many third-party libraries available for Python that can help you debug your code, such as pudb, which is a console-based debugger, and PySnooper, which allows you to easily log the values of variables and expressions during code execution.
Using these tools, developers can easily identify and fix errors in their code, leading to more efficient and effective software development.
How do you read error messages?
When debugging a Python program, error messages can provide valuable insights into the cause of the issue. Understanding how to read error messages is a critical skill that helps you identify and resolve problems more efficiently. Here’s a section on how to interpret error messages effectively:
Error messages in Python consist of several components that provide information about the encountered problem. By carefully analyzing these components, you can gain insights into the nature and location of the error.
First, pay attention to the error type mentioned at the beginning of the message. It indicates the category of the error, such as a syntax error, name error, type error, or value error. Knowing the error type helps you narrow down the possible causes of the issue.
Next, focus on the error message itself, which provides additional details about the error. It often describes what went wrong or provides specific information related to the error. Reading the error message can give you a better understanding of the root cause.
The traceback is a crucial part of the error message. It presents a stack trace that shows the sequence of function calls leading to the error. Each line in the traceback corresponds to a specific function call, with the most recent call appearing at the top. Analyzing the traceback allows you to trace the execution flow and identify the specific line of code where the error occurred. The traceback also includes the file path of the script where the error was encountered. This information is particularly useful in larger projects with multiple files, as it helps you locate the exact location of the error.
Make sure to note the line number mentioned in the error message. It indicates the line of code where the error occurred within the file. By referring to this line, you can quickly navigate to the problematic code and investigate further.
Pay attention to any additional contextual information provided in the error message. It may include nearby code lines or values involved in the error. Examining the context can provide clues about what might have caused the error.
To gain further insights, consider copying and pasting the error message into a search engine. Often, others have encountered similar errors, and online resources like forums, documentation, or Stack Overflow may offer explanations or solutions.
Developing the ability to read and interpret error messages effectively will significantly enhance your debugging skills. It allows you to quickly identify the problem area, understand the nature of the error, and take the necessary steps to resolve it.
How to reproduce and isolate bugs?
Reproducing and isolating bugs in Python is a crucial part of the debugging process. By creating minimal, self-contained examples and isolating the problematic code, you can identify the root cause of the bug and effectively resolve it. Let’s explore how to reproduce and isolate bugs using Python code examples:
Reproducing Bugs: To fix a bug, you need to be able to consistently reproduce it. Here’s an example of how to reproduce a bug:

In the above example, an empty list is passed to the calculate_average
function, resulting in a ZeroDivisionError. By running this code, you can consistently reproduce the bug and observe the error.
Isolating Bugs: Once you have reproduced the bug, the next step is to isolate it further to identify the root cause. Here are some techniques for isolating bugs:
- Binary Search: If the bug occurs in a large codebase, you can use a binary search approach to narrow down the problematic section. Comment out half of the code and see if the bug still persists. Based on the result, repeat the process on the relevant half until you identify the specific area causing the bug.
- Divide and Conquer: Break down the code into smaller sections and test them independently. By isolating specific portions, you can identify the precise area where the bug originates. Gradually reintegrate the sections to determine the exact combination that triggers the bug.
- Print Statements: Insert print statements at different points in your code to track the flow of execution and observe the intermediate values. By examining the output, you can identify the point at which the program deviates from the expected behavior.

Debugging Tools: Python provides various debugging tools, such as the built-in pdb
module or external libraries like pdbpp
and ipdb
. These tools allow you to set breakpoints, step through the code, inspect variables, and analyze the program’s execution flow.

By inserting the pdb.set_trace()
statement, you can pause the program’s execution at that point and interactively debug the code.
Unit Tests: Write comprehensive unit tests that cover different aspects of your code. By creating targeted tests, you can isolate specific functionalities and identify the conditions under which the bug occurs. A well-designed unit test can serve as a valuable tool for reproducing and isolating bugs.
By applying these techniques and utilizing appropriate tools, you can effectively reproduce and isolate bugs in your Python code. This process helps you pinpoint the root cause, leading to efficient and accurate bug fixes.
How do you use Logging and Error Handling?
Logging and error handling are crucial aspects of developing robust and reliable Python applications. They help you identify and handle errors gracefully, track the execution flow, and provide valuable information for debugging. Let’s explore logging and error handling in more detail:
Logging allows you to record important events, messages, and errors that occur during the execution of your program. It provides a way to track the program’s behavior, capture diagnostic information, and monitor its performance. The Python logging module provides a flexible and configurable logging system. Here’s an example of how to use logging:
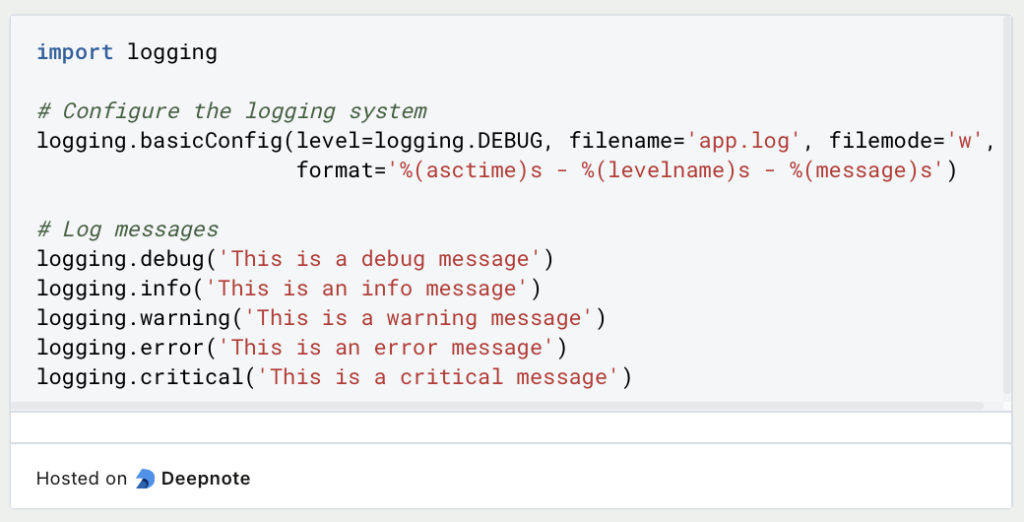
In the above example, we configure the logging system to write messages to a file named ‘app.log’. We set the log level to DEBUG, which includes all levels of messages. You can use different log levels (DEBUG, INFO, WARNING, ERROR, CRITICAL) to control the verbosity of the log output. By analyzing the log file, you can gain insights into the program’s execution and identify issues.
Error handling is the process of gracefully dealing with exceptions or errors that occur during the execution of your program. It allows you to handle and recover from errors, ensuring that your program doesn’t crash unexpectedly. The try-except block is commonly used for error handling in Python.

In the above example, we attempt to divide 10 by zero, which raises a ZeroDivisionError. By wrapping the code in a try block and specifying the except block for the specific exception, we can handle the error gracefully. You can catch different types of exceptions and perform appropriate actions, such as displaying an error message, logging the error, or taking corrective measures.
By combining logging and error handling techniques, you can effectively manage errors and exceptions in your Python code. Proper logging allows you to track events and diagnose issues, while error handling ensures that your program gracefully handles unexpected situations. These practices contribute to the stability and reliability of your applications.
This is what you should take with you
- Debugging is an essential skill for any programmer, and Python provides a wide range of tools and techniques to help you identify and fix issues in your code.
- Common tools for debugging in Python include print statements, logging, and debuggers like pdb and PyCharm.
- Techniques like error handling, testing, and code reviews can help prevent bugs from occurring in the first place.
- When debugging, it’s important to stay focused and methodical, starting with the most likely causes of the problem and ruling out possibilities one by one.
- Remember to use descriptive variable names, write clear comments, and keep your code organized to make it easier to debug in the first place.
- Finally, don’t be afraid to ask for help when you’re stuck – whether that means reaching out to a colleague or community forum or taking a break to clear your head and come back to the problem with fresh eyes.
What are Classes and Objects in Python?
Mastering Python's Object-Oriented Programming: Explore Classes, Objects, and their Interactions in our Informative Article!
What is Threading and Multiprocessing in Python?
Boost your Python performance and efficiency with threading and multiprocessing techniques. Learn how to harness parallel processing power.
What is Anaconda for Python?
Learn the essentials of Anaconda in Python for efficient package management and data science workflows. Boost your productivity today!
What are Regular Expressions?
Unlock powerful text manipulation in Python with regular expressions. Master patterns, syntax, and advanced techniques for data processing.
What is Object-Oriented Programming?
Master Object-Oriented Programming concepts in Python with our beginner's guide. Learn to create reusable code and optimize your coding skills.
What is Plotly?
Learn how to create interactive visualizations and dashboards with Plotly, a Python data visualization library.
Other Articles on the Topic of Debugging in Python
You can find the documentation of the Python Debugger here.