Python Try Except is a way to handle so-called exceptions in Python programs so that the application does not crash. With the help of Python Try Except, exceptions can be caught and handled.
What is the difference between Syntax Errors and Exceptions?
As we have probably all had to learn painfully, Python terminates a running program as soon as an error or exception occurs. An important distinction must be made here. The code can stop due to syntax errors, i.e. code that is simply written incorrectly and cannot be interpreted, or due to exceptions, i.e. syntactically correct code components that cause problems during execution.
A syntax error can be caused for example by wrongly set brackets:

Exceptions, on the other hand, are errors that occur during the execution of the application, even though the code is syntactically correct. This can happen, for example, when trying to add a string and a number together. The code itself is written correctly, but the implementation of this operation is not possible:
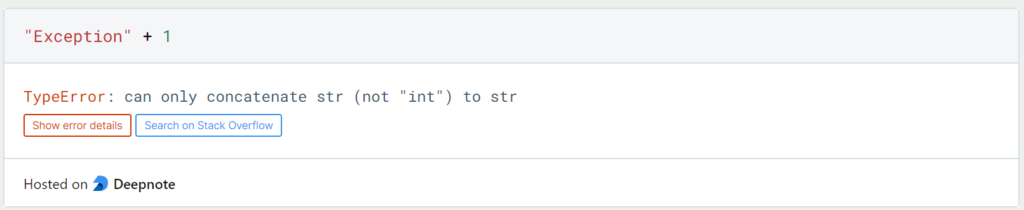
What are the types of exceptions in Python?
In Python, many different types of exceptions can occur when executing code. The following list describes the most common, but not all, types of exceptions:
AssertionError
: This exception occurs when the commandassert
was not used correctly or generates errors.ImportError
: If there are problems importing modules, you get anImport
Error. This can happen, for example, if a module, such as Pandas, has not yet been installed, incorrect or non-existent functions are to be loaded from a module, or the module with the specified name simply does not exist.IndexError
: When working with Python data objects that have an index, such as Python Tuples or Python Lists, anIndexError
can occur if indexes are used that cannot be found in the object.KeyError
: Similar to the IndexError, theKeyError
occurs when working with Python Dictionaries when a key could not be found in a Dictionary object.MemoryError
: When the machine’s memory is no longer sufficient to continue running the Python program, aMemoryError
occurs.UnboundLocalError
: When working with local variables, anUnboundLocalError
can occur as soon as a variable is referenced that has not yet been defined, i.e. that has not yet been assigned a value.
How does Python Try Except work?
The Python Try Except functionality makes it possible to deal with possible exceptions in a targeted manner by defining a routine that precisely describes how to handle the exception. This is especially useful if the probability of an exception is very high or an interruption of the program is to be prevented at all costs.
Two blocks are used for this purpose: Try and Except. The Try block is used to add the code that may cause exceptions during execution. If this code block runs without problems, the following Except block is simply skipped and the code is executed afterward. However, in case there is an exception in the Try block, the code in the Except block is automatically executed.

In this case, the addition of a
and b
is always executed, except when values are passed for the two variables that do not number. Then there would actually be a ValueError, but this is caught by our Python Try Except loop. Instead of the ValueError the text “a, b or both were not numbers. Please try again with different values”.
However, in a Python Try Except loop, you don’t necessarily have to specify the specific exception to respond to, it can also be defined to execute the Except block on any exception. In addition, a routine can be defined using finally
in case the program got by without an exception.

In which applications does it make sense to use Python Try Except?
In Data Science, it makes sense in many applications to use a Python Try Except loop to avoid terminating the program prematurely:
- Preparation of large Data Sets: If you want to prepare large datasets for Machine Learning to use as a training dataset, it is not uncommon for the preparation to take several hours. Depending on the quality of the data set, not all data types match the given ones. At the same time, you want to avoid the program stopping halfway through and you don’t notice it. For this, you can use a Python Try Except loop to simply skip individual records whose data quality is not correct and would lead to exceptions.
- Logging of Software: Here, too, the Python Try Except loop is needed, since the productive application should continue to work. Using the Except block, the error can be output to the logs and can then be evaluated. After the error has been fixed in a newer version, the new state can be deployed and ensures that the downtime of the application is as low as possible.
What are the best practices for using Python Try Except?
When using Python Try Except, you should follow some best practices to use it smoothly and get the most out of this statement.
- Be specific with the except statements: In most cases, it is best to only catch the specific exception types that are expected to occur. You should not use a general except statement to catch all possible exceptions. This makes the code more readable and easier to maintain.
- Use finally for cleanup code: The
finally
block is a good way to execute code that should always be executed, regardless of whether an exception occurred or not. For example, it can be used to close files or release other resources. - Avoid using try-except for the control flow: The Python try except statement is fundamentally different from conditional statements, such as the if statement. It should not be used for the control flow, i.e. to intercept certain cases. This can happen quickly, for example, if you want to prevent a division by zero. Such cases should be handled using conditional statements and not exceptions.
- Log errors for troubleshooting: Use the option in the except block to output and log important information for debugging purposes. This can be helpful for troubleshooting and can also shed light on the dark.
- Raise exceptions when appropriate: With the help of raise, you can also raise user-defined exceptions that are not included in the Python standard. Use these to trigger exceptional conditions that are unique to your program and should be handled.
- Use context managers for resource management: When working with resources, such as files, it can be more useful to work with context managers, such as the with statement, instead of exceptions. This ensures that resources are well managed and released when they are no longer needed.
With the help of these tips, your code can be made more robust and maintainable. They enable the sensible use of Python Try Except Anwe
What are Python tracebacks and how are they related to Python Try Except?
Although errors cannot be avoided in Python programming, there are ways of understanding how the error occurred and how it can be rectified as quickly as possible. The so-called exception tracebacks serve to provide an insight into what led to the error. They are detailed reports that show which line of code triggered the error and in which sequence of the function call problems occurred. Thus, the traceback helps you to find the exact location of the error and to understand the execution flow that led to the error.
When working with Python Try Except, these tracebacks are not relevant for the time being, as the try block is just trying to avoid the error. If an error occurs despite the use of Python Try Except, the traceback can be used to start a backtrace.
If an error occurs within a try block, Python does not stop directly but generates a structured list of the calls that took place before the error and ultimately led to the exception. This trace contains detailed information such as file names, line numbers, and function names. The function calls are listed from top to bottom so that the last line contains the code that ultimately led to the exception and is therefore a good starting point for troubleshooting.
By combining Python Try Except with the exception tracebacks, Python provides a good basis for debugging programming code. The traceback starts with the file path in which the error occurred. This can be particularly useful for more complex projects if modules are imported from other files.
The second piece of information is the line number in the file that could no longer be executed and led to the error. The last piece of information in this line is the function call that was mentioned in this line. Depending on the call, there may also be several tracebacks if the function is nested and has triggered several calls. The last line of the traceback then contains the error type and the error description.
This is what you should take with you
- Python Try Except is a way to handle so-called exceptions in Python programs so that the application does not crash.
- The Try Block encloses the lines that could potentially lead to exceptions. The Except Block, on the other hand, defines the code that should be executed in the event of an error. If no exception occurs, the code after the Python Try Except loop is simply executed.
- In addition, you can use the “finally” command to define what should happen if no error occurs in the Try Block.
What is Jenkins?
Mastering Jenkins: Streamline DevOps with Powerful Automation. Learn CI/CD Concepts & Boost Software Delivery.
What are Conditional Statements in Python?
Learn how to use conditional statements in Python. Understand if-else, nested if, and elif statements for efficient programming.
What is XOR?
Explore XOR: The Exclusive OR operator's role in logic, encryption, math, AI, and technology.
How can you do Python Exception Handling?
Unlocking the Art of Python Exception Handling: Best Practices, Tips, and Key Differences Between Python 2 and Python 3.
What are Python Modules?
Explore Python modules: understand their role, enhance functionality, and streamline coding in diverse applications.
What are Python Comparison Operators?
Master Python comparison operators for precise logic and decision-making in programming.
Other Articles on the Topic of Python Try Except
- At w3schools you can find a detailed article about Python Try Except.

Niklas Lang
I have been working as a machine learning engineer and software developer since 2020 and am passionate about the world of data, algorithms and software development. In addition to my work in the field, I teach at several German universities, including the IU International University of Applied Sciences and the Baden-Württemberg Cooperative State University, in the fields of data science, mathematics and business analytics.
My goal is to present complex topics such as statistics and machine learning in a way that makes them not only understandable, but also exciting and tangible. I combine practical experience from industry with sound theoretical foundations to prepare my students in the best possible way for the challenges of the data world.