Python functions are a fundamental concept in programming. They are reusable blocks of code that perform a specific task and return a value. Functions allow you to break down your code into smaller, more manageable pieces, making your code more modular and easier to read, understand, and debug. Python has a rich set of built-in functions, but it also allows you to create your own custom functions.
In this article, we will explore the basics of functions in Python, how to define and call them, and how to pass arguments and return values. We will also cover some advanced topics, such as lambda functions, recursion, and decorators.
How do you define a Python Function?
In Python, functions are defined using the def
keyword followed by the name and a pair of parentheses. The parentheses may contain optional parameters that the function can accept. The function definition is then followed by a colon (:) to indicate the start of the function’s code block. Be aware that after the def
keyword, the next line starts with an indentation to indicate the function code
The basic syntax of a function definition in Python is as follows:

The function name should follow the same naming conventions as variables, using lowercase letters and underscores for multiple words. Parameters are placeholders for values that can be passed into the function when it is called. They are listed inside the parentheses, separated by commas. Functions can have any number of parameters, including none.
Within the function code block, you write the statements that define the behavior of the function. These statements will be executed whenever the function is called. To indicate the end of the function code block, you simply dedent the statements. Unlike some other programming languages, Python does not use curly braces or explicit keywords to define the end of a function.
Optionally, a function can have a return statement to send a value back to the calling code. The return statement terminates the function and passes the specified value back to the caller. It’s important to note that a function can be defined before or after its usage in the code. However, it is recommended to define functions before they are called to maintain code readability.
What are the different types of arguments and how can you use them?
The first main building block of functions that we want to dig deeper into is the arguments or parameters. There are different types that we can use for different applications that we will explain in more detail in this section. In Python, functions can accept arguments or parameters that allow you to pass values into the function when it is called. Understanding different types of function arguments is essential for flexible and dynamic function usage.
- Positional Arguments: These are specified by their position in the function call. The order of the arguments matters as they are matched based on their position in the function definition. If we switch the order in the function call, then the age of 25 will be returned as name and the name will be returned as age.

- Keyword Arguments: They are identified by their parameter names in the function call. They allow you to specify arguments by name, which can be more readable and flexible. Furthermore, by giving the names of the parameters, the order does not matter.
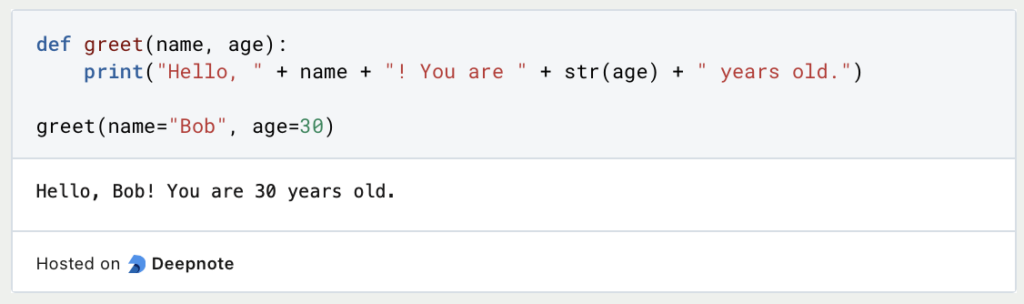
- Default Parameters: Functions can have default parameter values assigned. These values are used when an argument is not provided during the function call. Default parameters enable you to make certain parameters optional. Here’s an example:

- Variable-Length Arguments: Python allows functions to accept a variable number of arguments using
*args
and**kwargs
syntax.*args
allows passing multiple positional arguments, while**kwargs
allows passing multiple keyword arguments.

Understanding function arguments and parameters in Python enables you to create versatile and adaptable functions that can handle different inputs and scenarios. By leveraging positional arguments, keyword arguments, default parameters, and variable-length arguments, you can write functions that cater to a wide range of use cases.
How do you return values from a function?
In Python, functions can return values using the return
statement. Return values allow functions to provide output or results to the calling code. Understanding how to use return values is essential for capturing and utilizing the output of a function.
Here’s an example that demonstrates the usage of return values in a function:

In the example above, the add_numbers
function takes two arguments, a
and b
, and calculates their sum. The return
statement is used to send the computed sum back to the caller. The return value is then stored in the result
variable and printed, resulting in the output of 7
.
A function can return any valid Python object, including numbers, strings, lists, dictionaries, or even custom-defined objects. It can also return multiple values as a tuple, which can be unpacked by the calling code.
Here’s an example illustrating the return of multiple values:

In this case, the get_person_details
function returns three values: name
, age
, and city
. The function call assigns each returned value to a separate variable, which can be used independently in the calling code.
It’s important to note that a function can have multiple return
statements. Once a return
statement is encountered, the function execution stops, and the specified value is passed back to the caller. If a function does not have a return
statement or if the return
statement does not specify a value, the function returns None
by default.
What are the different lifetimes and scopes of functions?
In Python, the scope of a variable refers to its visibility and accessibility throughout the code. Understanding variable scope is crucial for managing and using variables effectively within functions and can prevent errors by keeping in mind which variable is valid.
- Local Variables: Variables defined inside a function have local scope, meaning they are only accessible within that function. Local variables are created when the function is called and destroyed when the function completes its execution.

In this example, the variable x
is defined within the my_function
function. It is accessible only inside the function, and attempting to access it outside the function will result in an error.
- Global Variables: Variables defined outside of any function or in the global scope are called global variables. They can be accessed from any part of the code, including within functions. To modify a global variable inside a function, you need to use the
global
keyword.

In this case, the global
keyword is used to indicate that the variable x
inside the function refers to the global variable x
. Therefore, modifying it within the function affects the global variable as well.
- Variable Lifetime: The lifetime of a variable is the period during which it exists in memory. For local variables, their lifetime starts when the function is called and ends when the function completes. Global variables, on the other hand, exist as long as the program is running. It’s important to note that when a function is called recursively, each recursive call creates a new set of local variables with its own scope and lifetime. Each recursive call has its independent set of variables, separate from the variables of other recursive calls.
Understanding scope and variable lifetime helps in preventing naming conflicts, organizing code, and managing data effectively. It’s generally recommended to use local variables within functions to encapsulate data and limit their visibility to where they are needed. Global variables should be used sparingly and with caution, as they can introduce complexity and make code harder to understand and maintain.
What are common built-in functions?
Python provides a wide range of built-in functions that are readily available for performing various operations. Understanding and utilizing these built-in functions can greatly simplify your code and enhance your programming experience. Here are some commonly used built-in functions in Python:
print()
: Outputs specified values or variables to the console.

len()
: Returns the length of an object, such as a string, list, or tuple.

input()
: Reads input from the user through the console.

type()
: Returns the type of an object.

range()
: Generates a sequence of numbers.

str()
,int()
,float()
,bool()
: Convert values to string, integer, float, or boolean types.

max()
,min()
: Returns the maximum or minimum value from a sequence or a set of arguments.

sum()
: Returns the sum of all elements in a sequence.

These are just a few examples of the many built-in functions available in Python. By utilizing these functions, you can perform a wide range of operations efficiently and effectively, saving time and effort in your programming tasks. Refer to the Python documentation for a comprehensive list of built-in functions and their detailed usage.
What is Recursion?
Recursion is a programming technique in which a function calls itself to solve a problem. Python supports recursive functions, and they can be used to solve problems that require iteration. Here is a section on recursion in Python:
Recursion is a powerful programming technique that allows a function to call itself. In Python, a function can be defined to be recursive by calling itself within the function body. When a function calls itself, it creates a new instance of itself that has its own set of variables and can execute its own code independently of the calling instance.
The process of recursion can be divided into two parts: the base case and the recursive case. The base case is the condition under which the function stops calling itself and returns a result. The recursive case is the condition under which the function calls itself to solve a subproblem.
Recursive functions are often used to solve problems that require iteration, such as searching, sorting, and traversing data structures. For example, the factorial function can be defined recursively as follows:

In this example, the base case is n == 0
, and the recursive case is n * factorial(n-1)
. The function calls itself with n-1
as the argument until the base case is reached.
Recursion can be an elegant solution to certain problems, but it has its limitations. Recursive functions can be slower and use more memory than iterative solutions, and they can also be more difficult to debug. It’s important to use recursion only when it is the best solution to a problem and to understand the limitations and trade-offs involved.
In summary, recursion is a powerful programming technique that allows a function to call itself to solve a problem. Python supports recursive functions, and they can be used to solve problems that require iteration. However, recursion can be slower and more memory-intensive than iterative solutions, and it’s important to use it only when it is the best solution to a problem.
What are Higher-Order Functions?
If you came to this point in the article, we can start dealing with more advanced topics namely higher-order functions, so functions that are taking other functions as input. This concept of treating functions as first-class objects allows for powerful and flexible programming paradigms. Here are some examples of higher-order functions in Python:
map()
: Applies a given function to each element of an iterable and returns an iterator of the results.

In this example, the map()
function takes the square()
function as its first argument and the numbers
list as the second argument. It applies the square()
function to each element of the numbers
list, resulting in a new list of squared numbers.
filter()
: Filters out elements from an iterable based on a given function that returns a boolean value.

Here, the filter()
function takes the is_even()
function as its first argument and the numbers
list as the second argument. It applies the is_even()
function to each element of the numbers
list and filters out the elements for which the function returns False
, resulting in a new list of even numbers.
reduce()
: Performs a cumulative computation on an iterable using a given function and returns a single value.

In this example, the reduce()
function takes the multiply()
function as its first argument and the numbers
list as the second argument. It applies the multiply()
function successively to the elements of the numbers
list, resulting in the cumulative product of all the elements.
Higher-order functions provide a powerful way to abstract and modularize code by encapsulating behavior in functions and passing them as arguments. By leveraging higher-order functions, you can write more concise, reusable, and expressive code in Python.
What are the Lambda Functions?
Python lambda functions are a concise way of creating small, anonymous functions that can be used in line with other code and are another example of higher-order functions. They are defined using the keyword lambda
, followed by a list of arguments and an expression that defines what the function should return.
Here is an example of a lambda function that takes two arguments and returns their sum:

This lambda function can be called like any other function, for example:

In this case, the variable result
would be assigned the value 7
.
Lambda functions can be especially useful when working with higher-order functions that take other functions as arguments, such as the built-in map
, filter
, and reduce
functions.
For example, here is how we can use a lambda function with map
to square each number in a list:

In this case, the variable squared
would be assigned a new list [1, 4, 9, 16, 25]
.
Lambda functions can also be used in a variety of other situations where a small, temporary function is needed. However, they should be used judiciously, as overly complex or nested lambda functions can be difficult to read and understand.
What are decorators in Python?
Decorators in Python are a powerful tool for modifying the behavior of functions or classes. A decorator is a function that takes another function as input and returns a new function that usually adds some extra functionality to the original function.
In Python, a decorator is applied to a function using the “@” symbol. This is followed by the name of the decorator function, which is placed above the function definition. Here’s an example of a simple decorator function:

In this example, the my_decorator
function is defined first. It takes a function as input and returns a new function, wrapper
, that adds some extra functionality to the original function.
The @my_decorator
syntax above the say_hello
function tells Python to apply the my_decorator
function to the say_hello
function.
Decorators can be used for many things, such as logging, timing functions, and caching results. They can also be used with classes to modify the behavior of methods.
Python decorators are a powerful feature that can help you write clean, readable code by separating concerns and making it easy to add extra functionality to your functions and classes.
This is what you should take with you
- Functions are an essential component of Python programming and enable code reusability and modularity.
- Defining a function in Python involves using the “def” keyword followed by a function name and a set of parentheses containing the arguments.
- Lambda functions, also known as anonymous functions, are a concise way to define simple functions in Python.
- Decorators in Python are functions that modify or enhance the functionality of other functions, without changing their code.
- Recursion is a technique where a function calls itself, allowing for the solution of a problem in terms of simpler versions of itself.
- Higher-order functions in Python can take other functions as arguments or return them as values, providing a powerful tool for functional programming.
- By understanding the various features and applications of functions in Python, you can write more efficient, modular, and reusable code.
What are Classes and Objects in Python?
Mastering Python's Object-Oriented Programming: Explore Classes, Objects, and their Interactions in our Informative Article!
What is Threading and Multiprocessing in Python?
Boost your Python performance and efficiency with threading and multiprocessing techniques. Learn how to harness parallel processing power.
What is Anaconda for Python?
Learn the essentials of Anaconda in Python for efficient package management and data science workflows. Boost your productivity today!
What are Regular Expressions?
Unlock powerful text manipulation in Python with regular expressions. Master patterns, syntax, and advanced techniques for data processing.
What is Object-Oriented Programming?
Master Object-Oriented Programming concepts in Python with our beginner's guide. Learn to create reusable code and optimize your coding skills.
What is Plotly?
Learn how to create interactive visualizations and dashboards with Plotly, a Python data visualization library.
Other Articles on the Topic of Functions in Python
The University of Stanford has an interesting article about functions in Python.