Space complexity is an important concept in computer science that refers to the amount of memory or storage required by an algorithm or program to solve a problem. It is closely related to time complexity, which measures the amount of time an algorithm takes to solve a problem. Space complexity is particularly important when dealing with large data sets or limited memory resources, such as in embedded systems or mobile devices.
This article will explore the concept of space complexity in more detail, including its definition, how it is measured, and its relevance to algorithm design and analysis.
What is Space Complexity?
Space complexity is a measure of how much memory or space an algorithm needs to execute. It is important to consider space complexity when dealing with large datasets or limited memory resources. The space complexity of an algorithm is typically expressed in terms of the amount of memory it uses relative to the size of its input.
There are different types of space complexity that can be used to evaluate algorithms:
- Auxiliary space complexity: This measures the extra space used by an algorithm, excluding the input space. For example, if an algorithm needs to create an additional data structure to solve a problem, the space required to store that data structure is considered the auxiliary space.
- Space complexity in terms of input size: This measures the space used by an algorithm as a function of the size of its input. In other words, it evaluates how much memory an algorithm needs as the input size grows.
- Total space complexity: This measures the total space used by an algorithm, including both the input space and any auxiliary space required.
It is important to note that space complexity is different from time complexity, which measures the amount of time an algorithm needs to execute as a function of its input size. Space and time complexity are often inversely proportional, meaning that an algorithm that uses less space may require more time to execute, and vice versa.
In general, algorithms with lower space complexity are preferable as they allow us to process larger inputs and can be executed more efficiently on devices with limited memory.
Why is Space Efficiency important?
The importance of space efficiency cannot be understated in software development. While often overshadowed by time efficiency, optimizing memory usage plays a critical role in achieving high-performance and scalable solutions. Space efficiency is crucial for several reasons.
Efficiently managing memory resources allows programs to operate smoothly, even when dealing with large datasets or limited memory environments. By minimizing unnecessary memory consumption, programs can handle more significant workloads and reduce the risk of memory-related issues such as crashes or slowdowns.
Space-efficient programs are more scalable, accommodating growing data sizes without exhausting memory resources. This scalability is vital in today’s data-driven world, where information volume continues to expand rapidly. Designing space-efficient algorithms and data structures enables applications to handle increasing data sizes efficiently and remain responsive.
Optimizing space usage improves performance. Efficient memory utilization reduces time spent on memory allocation, deallocation, and garbage collection. This results in faster execution, reduced latency, and improved overall responsiveness. Space efficiency is especially crucial in performance-critical applications like real-time systems or high-throughput data processing.
Space efficiency can also translate into cost savings, particularly in cloud computing or server environments where resources are allocated and billed based on usage. By minimizing memory footprint, organizations can reduce infrastructure costs, optimize resource allocation, and lower operational expenses.
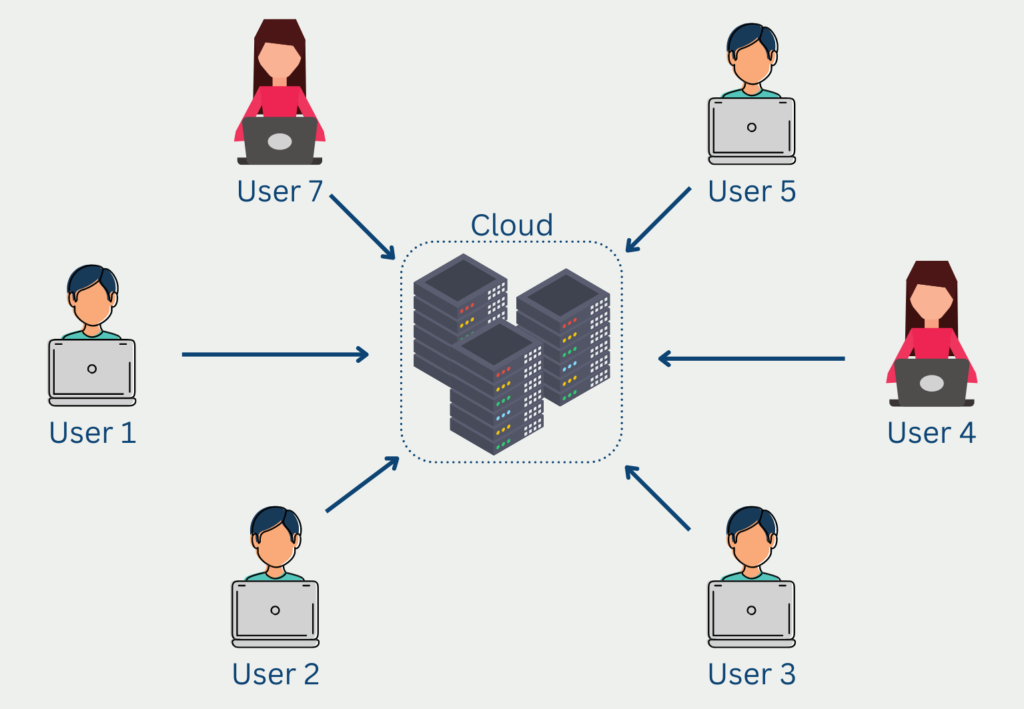
In resource-constrained environments like mobile devices or embedded systems, space efficiency is vital. These devices often have limited memory capacities, necessitating space-efficient programming to ensure optimal performance, minimize power consumption, and provide a smooth user experience.
Space efficiency is crucial in data-intensive applications such as data analysis, Machine Learning, or big data processing. Efficient memory management is required to avoid bottlenecks, enable faster computations, and handle complex analytics tasks.
Beyond performance and cost savings, space efficiency also contributes to environmental sustainability. By reducing memory consumption, energy consumption can be minimized, resulting in lower carbon footprints and a more eco-friendly computing ecosystem.
Considering the importance of space efficiency is vital for building robust and efficient software solutions in today’s data-intensive and resource-constrained environments. By emphasizing optimized memory utilization, developers can create lean, scalable, and high-performing applications.
How to measure the Space Complexity?
Measuring space complexity is essential to evaluate the efficiency of an algorithm in terms of memory usage. The space complexity of an algorithm is usually measured in terms of the amount of memory required to store the input data and the intermediate results of the algorithm. The actual amount of memory required by an algorithm can depend on many factors, including the size of the input, the data structures used by the algorithm, and the number of recursive calls made by the algorithm.
There are several ways to measure space complexity, including:
- Worst-case space complexity: This measures the maximum amount of memory required by an algorithm for any possible input. This is often the most useful measure of space complexity since it provides an upper bound on the memory requirements of the algorithm.
- Average-case space complexity: This measures the average amount of memory required by an algorithm over all possible inputs. This can be more difficult to compute than worst-case complexity since it requires knowledge of the input distribution.
- Best-case space complexity: This measures the minimum amount of memory required by an algorithm for any possible input. This is often not a useful measure of space complexity since it does not provide an upper bound on the memory requirements of the algorithm.
- Amortized space complexity: This measures the average amount of memory required by an algorithm over a sequence of operations. This is useful for algorithms that perform a series of operations on a data structure, where the memory requirements of each operation may depend on the previous operations.
It is important to note that the space complexity of an algorithm can depend on the machine used to execute the algorithm. Different machines may have different amounts of memory available or different ways of allocating and managing memory. Therefore, the space complexity of an algorithm is usually expressed in terms of the order of growth of the memory requirements as the input size increases, rather than in terms of absolute memory usage.
To measure the space complexity, follow these steps:
- Identify the Variables and Data Structures: Analyze the algorithm and identify the variables and data structures used to store and manipulate data. This includes arrays, lists, matrices, stacks, queues, etc.
- Assign Space Values: Determine the space occupied by each variable or data structure. For example, an integer typically occupies a fixed amount of memory, while an array’s space depends on its size and the type of elements it holds.
- Analyze Iterations and Recursive Calls: Consider loops, iterations, and recursive calls within the algorithm. Determine if any new variables or data structures are created during each iteration or recursive call, and account for their space requirements.
- Calculate Total Space Complexity: Sum up the space requirements of all variables, data structures, and input space to calculate the total space complexity. This provides an estimate of the memory required by the algorithm as a function of the input size.
What are the trade offs between Space and Time Complexity?
The trade-off between space and time complexity is a fundamental consideration in algorithm design and optimization. It involves making strategic decisions on how to allocate computational resources between memory usage and execution time. Balancing these two aspects is crucial to achieve optimal algorithm performance and efficiency.
Space complexity refers to the amount of memory required by an algorithm to solve a problem. It measures how the memory usage grows as the input size increases. Space-efficient algorithms aim to minimize memory consumption, utilizing only the necessary storage to perform computations. This can be achieved through techniques like reusing memory, discarding unnecessary data, or employing data structures that optimize memory usage.
Time complexity, on the other hand, focuses on the computational efficiency of an algorithm, particularly the time required to execute it. It describes how the execution time increases as the input size grows. Time-efficient algorithms aim to minimize the number of operations or iterations needed to solve a problem, enabling faster execution.
The trade-off between space and time complexity arises because reducing one often comes at the expense of the other. For example, using additional memory to store precomputed values or caching results can improve time efficiency but increases space requirements. Conversely, reducing memory usage by recalculating values on-the-fly may improve space efficiency but can lead to longer execution times.
The choice between space and time optimization depends on the specific requirements of the problem and the constraints of the system. In scenarios with limited memory resources, prioritizing space efficiency may be necessary to ensure the algorithm can run within the available memory limits. On the other hand, in situations where speed is critical, optimizing time complexity becomes paramount, even if it requires increased memory usage.
It’s important to note that the trade-off is not always linear, and different algorithms may exhibit different trade-off characteristics. Some algorithms may offer a fine-grained control over the trade-off, allowing developers to adjust the balance based on their specific needs.
The optimal balance between space and time complexity depends on various factors, such as the problem’s input size, available memory, computational power, and desired performance goals. It requires careful analysis, benchmarking, and profiling to identify the most suitable approach.
Overall, understanding and managing the trade-off between space and time complexity is essential for designing efficient algorithms. Striking the right balance allows developers to achieve optimal performance, reduce resource consumption, and meet the requirements of the problem at hand.
Which factors affect Space Complexity?
Space complexity refers to the amount of memory used by an algorithm during its execution. There are several factors that can affect the space complexity of an algorithm, including:
- Input size: The amount of memory required by an algorithm can be proportional to the size of the input data. For example, if an algorithm processes a large dataset, it will need more memory to store the data and intermediate results.
- Data structures: The choice of data structures can also affect the space complexity of an algorithm. Some data structures require more memory than others to store the same data. For example, an array may require more memory than a linked list to store the same number of elements.
- Auxiliary memory: The use of auxiliary memory, such as disk space or external memory, can also affect the space complexity of an algorithm. If an algorithm needs to store large amounts of data that cannot fit in main memory, it may need to use auxiliary memory, which can be slower and more expensive than main memory.
- Recursion: Recursive algorithms can also affect the space complexity of an algorithm. Each recursive call requires additional memory to store the call stack, which can quickly consume a large amount of memory for deep recursion levels.
- Temporary variables: The use of temporary variables, such as counters or flags, can also affect the space complexity of an algorithm. Each variable requires a certain amount of memory, and using too many temporary variables can increase the overall space complexity of an algorithm.
Measuring the space complexity of an algorithm is usually done by analyzing the amount of memory used by the algorithm as a function of the input size. The space complexity is often expressed in terms of the big O notation, which provides an upper bound on the amount of memory used by the algorithm for large input sizes.
Which techniques can reduce the Space Complexity?
Optimizing space complexity is an important aspect of algorithm design. Here are some strategies for reducing space complexity:
- Use in-place algorithms: In-place algorithms modify the input data structure without using any additional space. This approach is efficient when the input data is large and copying it takes a lot of memory.
- Choose appropriate data structures: Selecting the right data structure for a problem can significantly reduce the memory usage. For example, if the order of elements does not matter, then using a set or a hash table can reduce the space requirements.
- Use dynamic programming: Dynamic programming is an algorithmic technique that reduces the time and space complexity of a problem by breaking it down into smaller subproblems. The results of the subproblems are stored in a table to avoid recomputation.
- Implement lazy evaluation: Lazy evaluation is a programming technique that delays the evaluation of an expression until it is actually needed. This approach can save memory because it avoids the computation and storage of unnecessary values.
- Use streaming algorithms: Streaming algorithms process data sequentially and only store a small amount of data in memory at a time. This approach is useful for processing large datasets that do not fit into memory.
- Implement garbage collection: Garbage collection is a memory management technique used by many programming languages to automatically deallocate memory that is no longer needed. This can reduce the memory usage of an algorithm by freeing up memory that would otherwise be used by unused data structures.
By using these strategies, it is possible to reduce the space complexity of an algorithm and make it more efficient in terms of memory usage. However, it is important to balance space complexity with other factors, such as time complexity and code readability, to ensure that the algorithm is optimal for the given problem.
What are challenges and limitations of Space Complexity?
Despite the importance of space complexity in algorithm analysis, there are several challenges and limitations associated with it. Some of these include:
- Trade-offs between space and time complexity: In some cases, optimizing the space complexity of an algorithm can lead to an increase in its time complexity and vice versa. This trade-off can make it challenging to design algorithms that are both space and time-efficient.
- Difficulty in accurately measuring space complexity: Measuring space complexity can be a complex task, especially when dealing with algorithms that use dynamic memory allocation or when the amount of memory used varies depending on the input size.
- Hardware constraints: The amount of available memory on a computer or other computing device can limit the size of the input that an algorithm can handle. This limitation can make it challenging to design algorithms that can handle very large datasets.
- Algorithmic limitations: Some algorithms are inherently space-intensive, and it may be difficult to reduce their space complexity without sacrificing their performance.
- The complexity of the problem itself: In some cases, the space complexity of an algorithm may be limited by the complexity of the problem it is trying to solve. For example, some problems may require the storage of large amounts of data, which can make it challenging to design algorithms with low space complexity.
- Incomplete understanding of the problem: In some cases, it may be challenging to fully understand the problem being solved and its requirements, making it difficult to design algorithms with optimal space complexity.
Overall, managing space complexity is an essential aspect of algorithm design, and understanding the challenges and limitations associated with it can help designers create more efficient and effective algorithms.
This is what you should take with you
- Space complexity is an important consideration in algorithm design and analysis.
- It refers to the amount of memory an algorithm requires to run.
- Space complexity can be measured in terms of the number of bits, bytes, or words needed by an algorithm.
- The space complexity of an algorithm is affected by various factors such as data structures, recursion, and input size.
- Optimizing space complexity can involve strategies such as reducing memory usage, implementing more efficient data structures, and avoiding redundant calculations.
- There are several challenges and limitations to optimizing space complexity, including trade-offs between time and space, hardware limitations, and the inherent complexity of certain algorithms.
What is Jenkins?
Mastering Jenkins: Streamline DevOps with Powerful Automation. Learn CI/CD Concepts & Boost Software Delivery.
What are Conditional Statements in Python?
Learn how to use conditional statements in Python. Understand if-else, nested if, and elif statements for efficient programming.
What is XOR?
Explore XOR: The Exclusive OR operator's role in logic, encryption, math, AI, and technology.
How can you do Python Exception Handling?
Unlocking the Art of Python Exception Handling: Best Practices, Tips, and Key Differences Between Python 2 and Python 3.
What are Python Modules?
Explore Python modules: understand their role, enhance functionality, and streamline coding in diverse applications.
What are Python Comparison Operators?
Master Python comparison operators for precise logic and decision-making in programming.
Other Articles on the Topic of Space Complexity
You can find an interesting article on the topic on GitHub.

Niklas Lang
I have been working as a machine learning engineer and software developer since 2020 and am passionate about the world of data, algorithms and software development. In addition to my work in the field, I teach at several German universities, including the IU International University of Applied Sciences and the Baden-Württemberg Cooperative State University, in the fields of data science, mathematics and business analytics.
My goal is to present complex topics such as statistics and machine learning in a way that makes them not only understandable, but also exciting and tangible. I combine practical experience from industry with sound theoretical foundations to prepare my students in the best possible way for the challenges of the data world.