Welcome to our comprehensive guide on regular expressions in Python. Regular expressions are a powerful tool for pattern matching and text manipulation, allowing you to search, extract, and manipulate strings of text with precision. Whether you’re a beginner or an experienced Python programmer, understanding regular expressions can greatly enhance your text processing capabilities.
In this article, we will explore the fundamentals of regular expressions in Python, learn how to construct patterns, utilize various metacharacters and quantifiers, and leverage the re
module to apply it in practical scenarios.
What are Regular Expressions?
Regular expressions in Python are powerful tools for pattern matching and text manipulation. They provide a concise and flexible way to search, extract, and replace specific patterns within strings of text. Regular expressions are a sequence of characters that define a search pattern, which can include a combination of letters, digits, special characters, and metacharacters.
In Python, regular expressions are supported through the re
module, which provides a set of functions and methods. You can perform tasks like validating input, extracting specific information from text, performing advanced text processing, and much more. Regular expressions offer a versatile and efficient solution for various text-related challenges in Python programming.
How to find basic patterns?
Regular expressions provide a powerful and flexible way to search, match, and manipulate text patterns in Python. Let’s explore some common syntax and basic patterns with code examples:
- Literal Matching: Use specific strings to find exact matches.
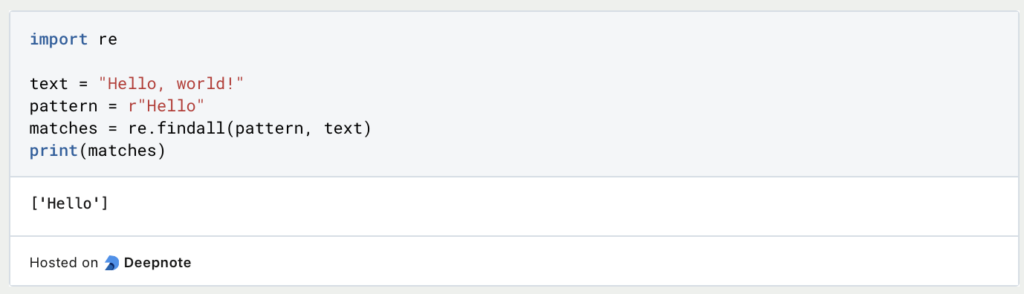
In this example, the regular expression Hello
matches the exact string “Hello” in the given text.
- Character Classes: Match any character from a set using square brackets.
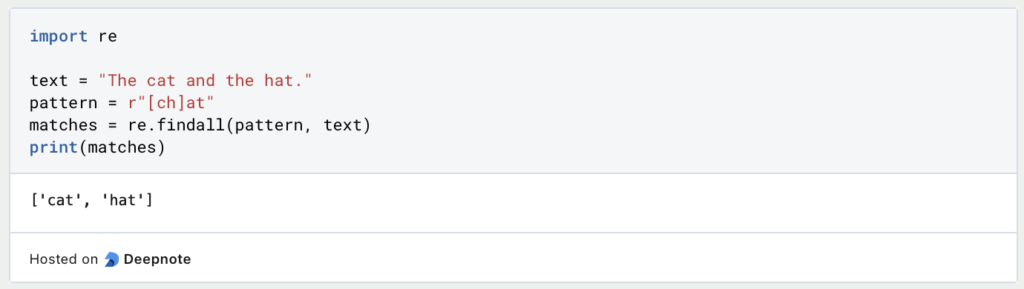
Here, the pattern [ch]at
matches either “cat” or “hat” as the character class [ch]
allows for either “c” or “h”.
- Wildcard: Match any character (except a newline) using a dot (
.
).
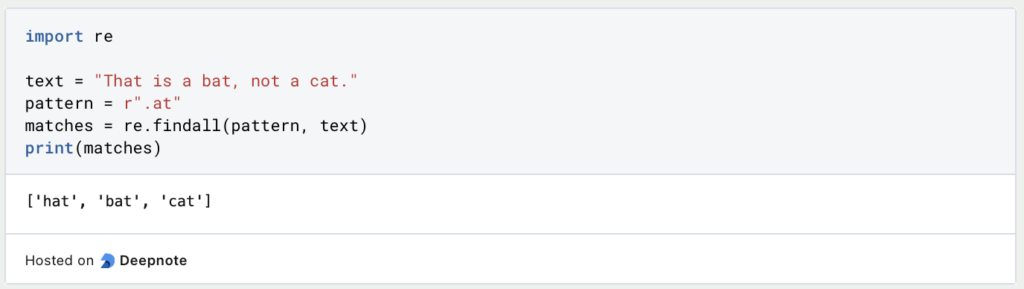
The pattern .
matches any character, and at
matches “at” following any character.
- Quantifiers: Match multiple occurrences of a pattern.
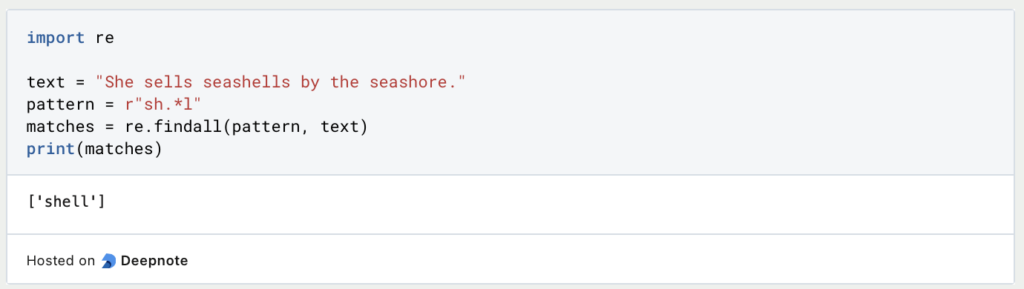
In this example, sh
matches “sh” and .*
matches any character (.
) zero or more times (*
), followed by “l”.
- Anchors: Match at the beginning or end of a line.
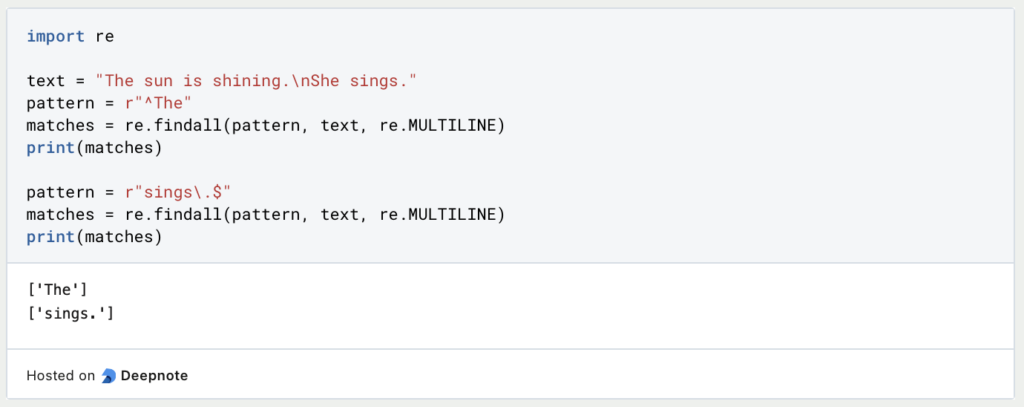
The pattern ^The
matches “The” at the beginning of a line, while sings\.$
matches “sings.” at the end of a line ($
). The re.MULTILINE
flag enables multiline matching.
These examples demonstrate the basic syntax and patterns in regular expressions. With regular expressions, you can perform advanced text manipulation tasks efficiently in Python.
How do you use Matching and Searching?
In Python, the re
module provides functions to match and search for patterns using regular expressions. Let’s explore how to perform matching and searching operations with examples:
- Match: Use the
match()
function to check if a pattern matches at the beginning of a string.
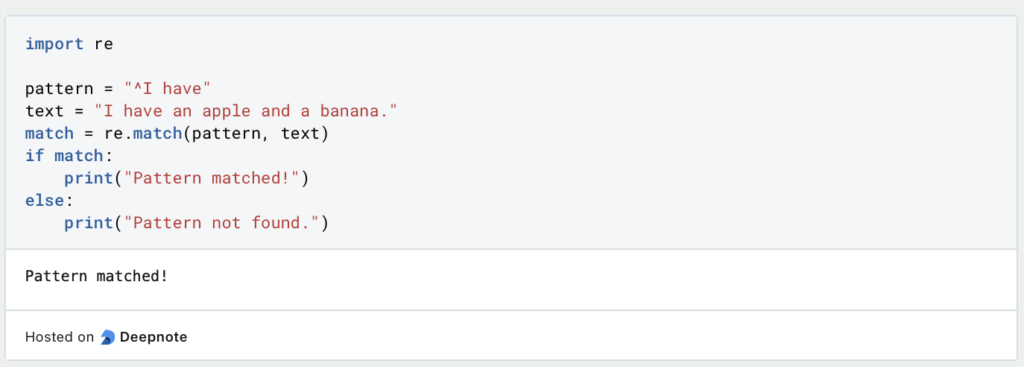
In this example, the pattern I have
is matched at the beginning of the string, so the output will be “Pattern matched!”.
- Search: Use the
search()
function to find the first occurrence of a pattern in a string.
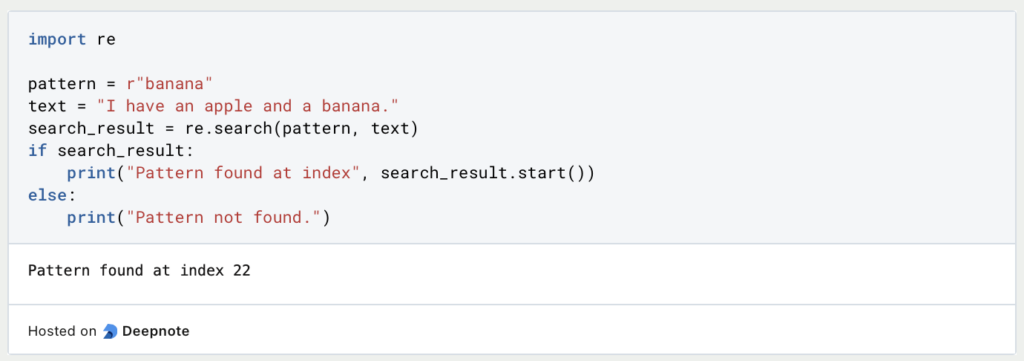
Here, the pattern banana
is found in the string, and the output will be “Pattern found at index 22”.
- Find All: Use the
findall()
function to find all occurrences of a pattern in a string.
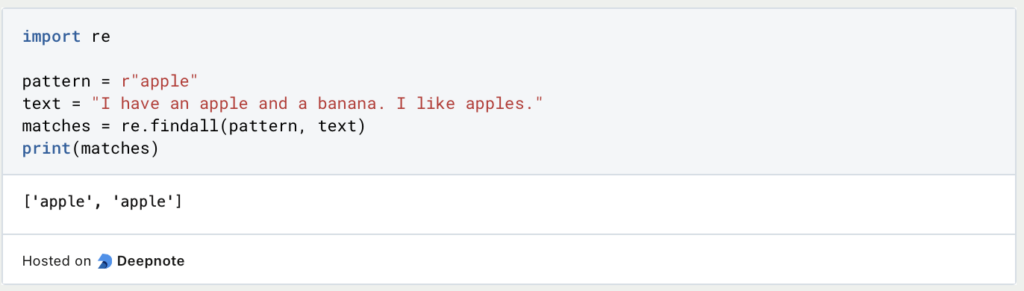
In this example, the pattern apple
is found twice in the string, and the output will be ['apple', 'apple']
.
- Find Iter: Use the
finditer()
function to find all occurrences of a pattern and iterate over the match objects.
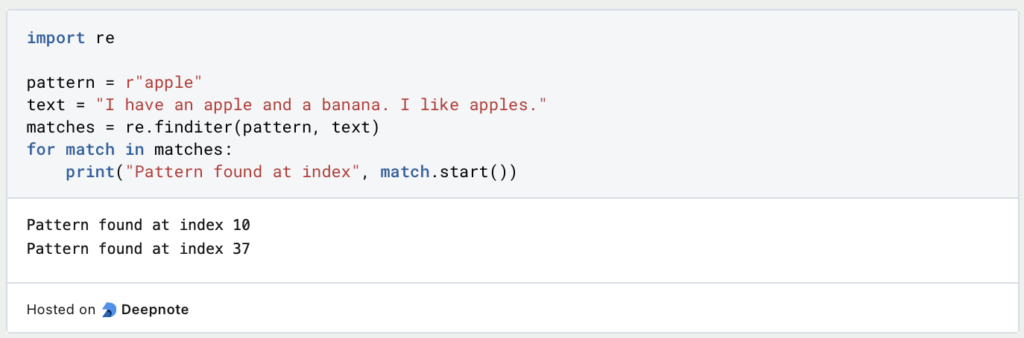
Here, the pattern apple
is found twice, and the start index of each match is printed.
These examples demonstrate how to perform matching and searching operations in Python using regular expressions. The re
module provides powerful functionality to work with patterns and manipulate text effectively.
What are Metacharacters and Special Sequences?
In regular expressions, meta characters and special sequences are used to define patterns with specific meanings. These elements enhance the flexibility and power of regular expressions. Let’s explore some commonly used meta characters and special sequences with examples:
- Meta Characters:
.
(dot): Matches any single character except a newline.^
(caret): Matches the start of a string.$
(dollar sign): Matches the end of a string.[]
(square brackets): Matches any single character within the brackets.|
(pipe): Acts as an OR operator, allowing multiple alternatives in a pattern.()
(parentheses): Groups patterns and captures matched content.
Example:
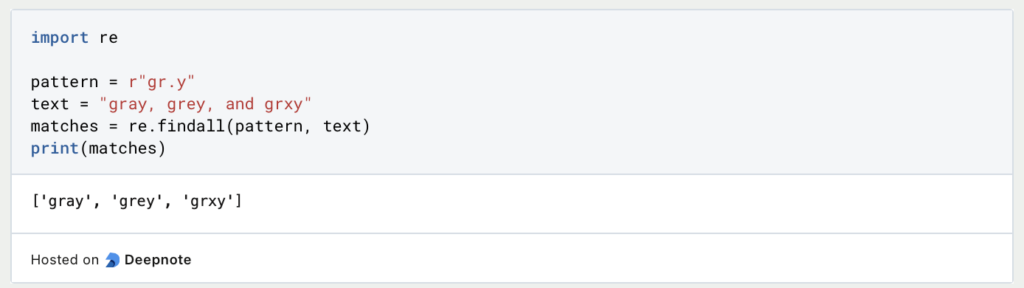
In this example, the pattern gr.y
matches words that have ‘gr’ followed by any character and then ‘y’. It matches ‘gray’ and ‘grey’ and ‘grxy’.
- Special Sequences:
\d
: Matches any digit (equivalent to[0-9]
).\w
: Matches any alphanumeric character (equivalent to[a-zA-Z0-9_]
).\s
: Matches any whitespace character (spaces, tabs, newlines).\b
: Matches a word boundary.\A
: Matches only at the start of a string.\Z
: Matches only at the end of a string.
Example:
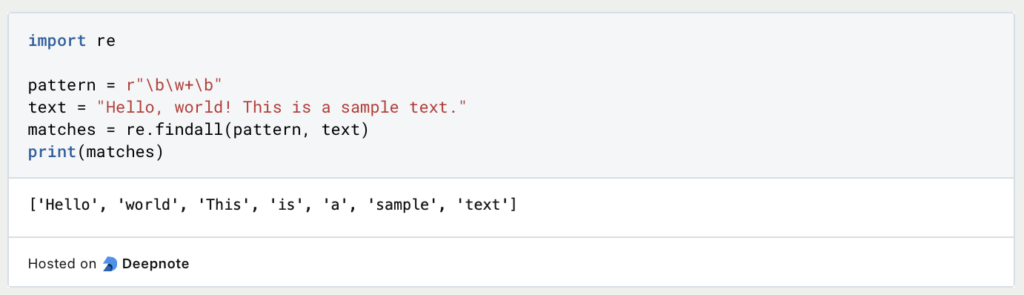
In this example, the pattern \b\w+\b
matches whole words in the text. It extracts ‘Hello’, ‘world’, ‘This’, ‘is’, ‘a’, ‘sample’, and ‘text’.
Understanding meta characters and special sequences allows for more precise pattern matching and manipulation of text using regular expressions in Python. Experiment with different combinations to build powerful and versatile patterns for your specific needs.
How do you use Grouping and Capturing?
In regular expressions, grouping and capturing allow you to specify and extract specific portions of a matched pattern. Grouping is done using parentheses ()
and is useful for applying quantifiers and modifiers to a group of characters. Capturing allows you to extract the matched content of specific groups. Let’s explore how grouping and capturing work with some examples:
Example 1: Grouping with Quantifiers
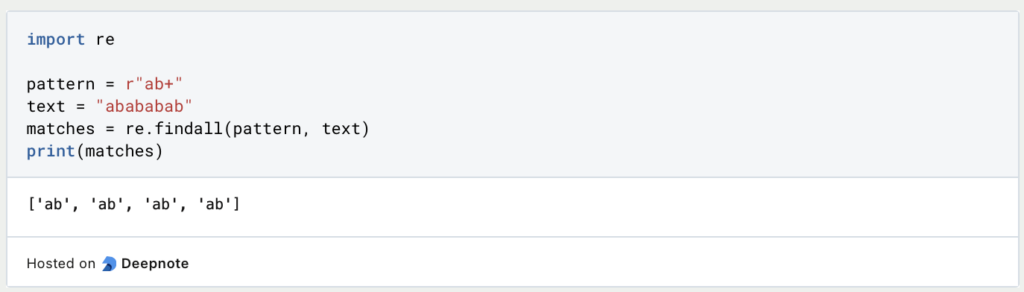
In this example, the pattern (ab)+
matches one or more occurrences of the group ab
. It captures and returns each matched group as a separate element in the result.
Example 2: Capturing with Parentheses
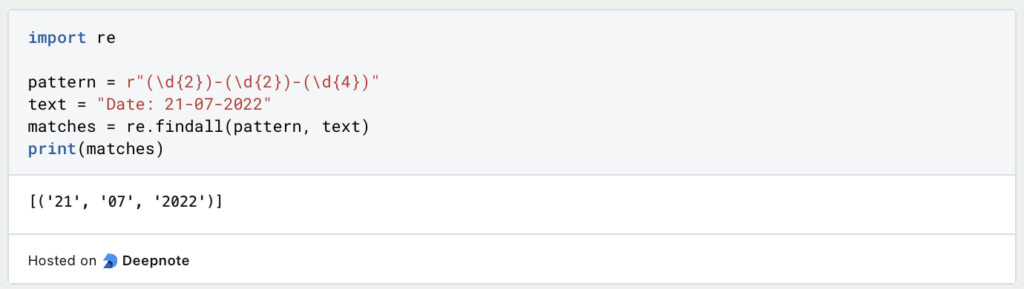
In this example, the pattern (\d{2})-(\d{2})-(\d{4})
captures groups of two digits separated by hyphens. The matched groups (’21’, ’07’, ‘2022’) are returned as a tuple.
Grouping and capturing allow you to structure and extract specific parts of the matched text. They are essential for performing more complex pattern matching and data extraction tasks. By using parentheses to define groups and accessing the captured content, you can achieve more precise and targeted results in your regular expression operations.
How do you use Modifiers and Flags?
Modifiers and flags in regular expressions are special options that modify the behavior of the pattern matching process. They allow you to control aspects such as case sensitivity, multiline matching, and the interpretation of special characters. Let’s explore some commonly used modifiers and flags in Python:
- Case Insensitivity (re.I):
There.I
flag enables case-insensitive matching. It allows the pattern to match regardless of whether the characters are uppercase or lowercase. Example:
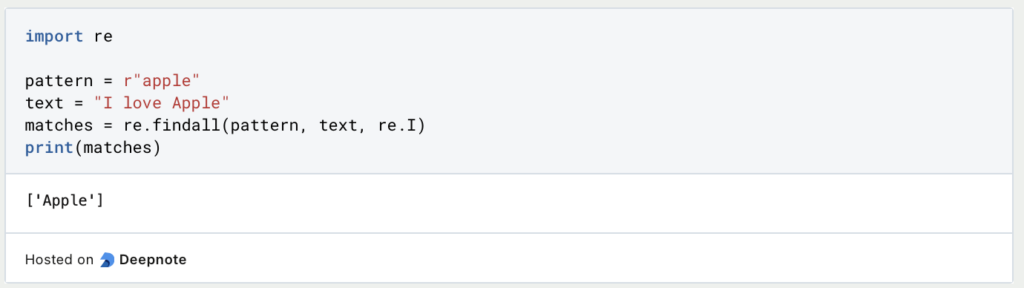
- Multiline Matching (re.M):
There.M
flag enables multiline matching. It allows the pattern to match across multiple lines, considering the start (^
) and end ($
) anchors for each line. Example:
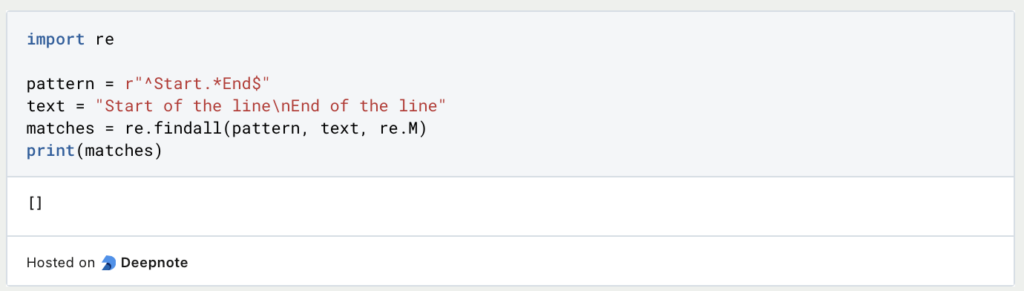
- Dot All Matching (re.S):
There.S
flag enables dot-all matching. It allows the dot (.
) metacharacter to match any character, including newline characters (\n
). Example:
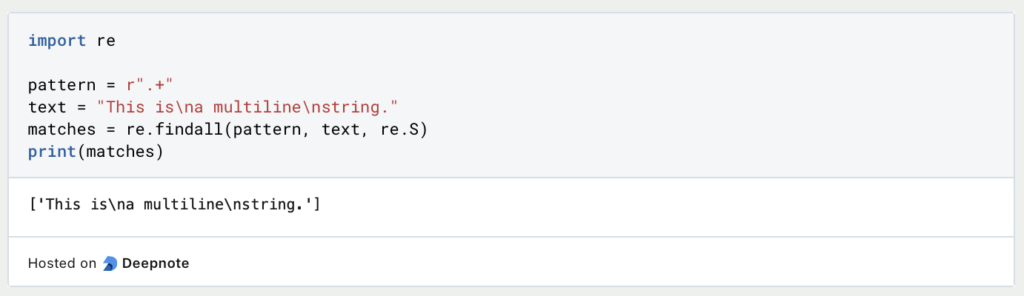
Modifiers and flags provide additional flexibility and control over regular expression matching. By applying the appropriate modifiers, you can customize the behavior of the pattern matching process to suit your specific requirements.
What are common applications of using Regular Expressions?
Regular expressions are widely used in various domains to perform pattern matching and text manipulation tasks. Here are some common applications of regular expressions:
- Data Validation: Regular expressions are often used to validate and enforce data input formats. They can ensure that user-provided data, such as email addresses, phone numbers, or credit card numbers, adhere to specific patterns and criteria.
- Text Search and Extraction: Regular expressions enable efficient text search and extraction operations. They can be used to find specific patterns or keywords within a document, extract relevant information from unstructured text, or parse log files for specific data points.
- Data Cleaning and Transformation: Regular expressions are valuable for data cleaning and transformation tasks. They can help remove unwanted characters, correct formatting inconsistencies, and extract specific information from raw data. For example, you can use regular expressions to clean up messy datasets or convert data from one format to another.
- Web Scraping: Regular expressions play a crucial role in web scraping, where data is extracted from websites. They can be used to locate and extract specific HTML elements or patterns from web pages, allowing for automated data extraction and analysis.
- Text Processing and Natural Language Processing (NLP): Regular expressions are vital in text processing and NLP tasks. They can assist in tokenization, stemming, removing stopwords, identifying sentence boundaries, and performing various linguistic analyses on textual data.
- Code Refactoring: Regular expressions are valuable tools for code refactoring tasks. They can help automate search and replace operations, making it easier to modify code patterns, update variable names, or refactor large codebases efficiently.
- Syntax Highlighting and Lexical Analysis: Regular expressions are commonly used in text editors and programming tools for syntax highlighting and lexical analysis. They can identify and highlight different elements of code, such as keywords, strings, comments, and variables, based on predefined patterns.
Regular expressions provide a powerful and flexible way to work with text data. Their applications span multiple fields, from data validation and manipulation to web scraping, text processing, and code refactoring. By mastering regular expressions, you can enhance your ability to handle complex text-related tasks effectively.
This is what you should take with you
- Regular expressions are powerful tools for pattern matching and text manipulation.
- They find applications in data validation, text search, data cleaning, web scraping, and more.
- Regular expressions enable efficient text processing and code refactoring.
- They are widely used in fields such as data analysis, natural language processing, and web development.
- By mastering regular expressions, you can enhance your ability to handle complex text-related tasks effectively.
- Regular expressions are an essential skill for anyone working with textual data in Python or other programming languages.
Thanks to Deepnote for sponsoring this article! Deepnote offers me the possibility to embed Python code easily and quickly on this website and also to host the related notebooks in the cloud.
What are Classes and Objects in Python?
Mastering Python's Object-Oriented Programming: Explore Classes, Objects, and their Interactions in our Informative Article!
What is Threading and Multiprocessing in Python?
Boost your Python performance and efficiency with threading and multiprocessing techniques. Learn how to harness parallel processing power.
What is Anaconda for Python?
Learn the essentials of Anaconda in Python for efficient package management and data science workflows. Boost your productivity today!
What is Object-Oriented Programming?
Master Object-Oriented Programming concepts in Python with our beginner's guide. Learn to create reusable code and optimize your coding skills.
What is Plotly?
Learn how to create interactive visualizations and dashboards with Plotly, a Python data visualization library.
What is Matplotlib?
Visualize your data like a pro with Matplotlib: A comprehensive guide to the Python plotting library.
Other Articles on the Topic of Regular Expressions
This link will get you to my Deepnote App where you can find all the code that I used in this article and can run it yourself.