In today’s world, software applications are built to interact with each other in a seamless and efficient manner. This has given rise to the need for standardized ways of exchanging data between applications over the Internet. RESTful APIs (Representational State Transfer APIs) have emerged as the industry standard for web-based APIs due to their simplicity, scalability, and flexibility. In this article, we will explore the fundamentals of RESTful APIs, their architecture, and how they are used in modern web development.
What are RESTful APIs?
RESTful APIs are a type of web service that is designed to allow different applications to communicate with one another over the internet. The term “REST” stands for “Representational State Transfer”, and is a design principle that has been used in web development for many years. The idea behind RESTful APIs is to create a standardized way for different applications to communicate with one another, regardless of the programming language or platform they are built on.
How do RESTful APIs work?
At their core, RESTful APIs are designed to be simple and easy to use. They rely on a few basic HTTP methods (GET, POST, PUT, DELETE) to allow different applications to interact with one another. When an application wants to access data from another application, it sends a request to the API using one of these methods. The API then processes the request, retrieves the requested data, and sends it back to the requesting application in a standardized format (usually JSON or XML).
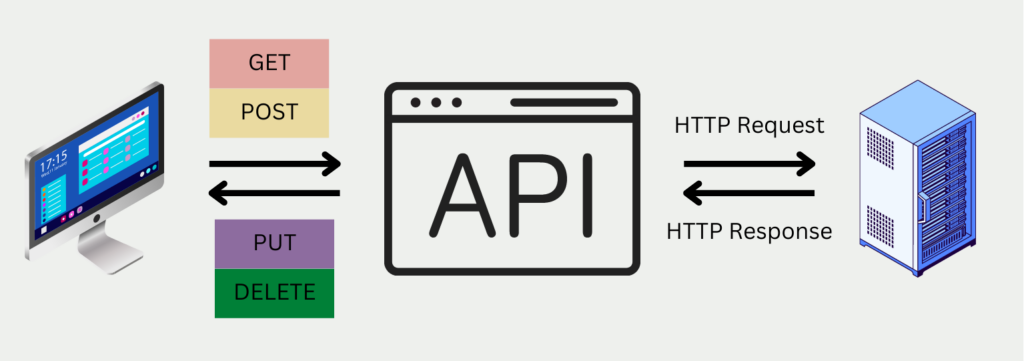
Which applications use RESTful APIs?
Real-world applications using RESTful APIs have been expanding rapidly in recent years, with many organizations utilizing this technology to improve their operations and services. Some notable examples include:
- Social Media Platforms: RESTful APIs are used by popular social media platforms like Twitter, Facebook, and Instagram to allow developers to access their platform’s features, such as posting tweets, retrieving user data, and managing user accounts.
- E-Commerce: Many online retailers use RESTful APIs to integrate their platforms with payment gateways and shipping services, providing a streamlined checkout process for customers.
- Banking and Finance: RESTful APIs are used in the financial sector to provide services such as account balance inquiries, fund transfers, and investment management.
- Healthcare: RESTful APIs are used in healthcare applications to provide access to patient data, electronic health records, and medical research databases.
- IoT: RESTful APIs are used to connect Internet of Things (IoT) devices to the Internet, enabling them to communicate with each other and with cloud-based services.
- Gaming: RESTful APIs are used in the gaming industry to provide services such as in-game purchases, user account management, and matchmaking.
Overall, RESTful APIs have become a key technology for building scalable, flexible, and interoperable applications in a wide range of industries.
Why are RESTful APIs so important?
RESTful APIs are important for a number of reasons. First and foremost, they allow different applications to communicate with one another in a standardized way. This means that developers can build applications that work together seamlessly, regardless of the programming language or platform they are built on. This is especially important in today’s world of cloud computing, where applications are often distributed across multiple servers and platforms.
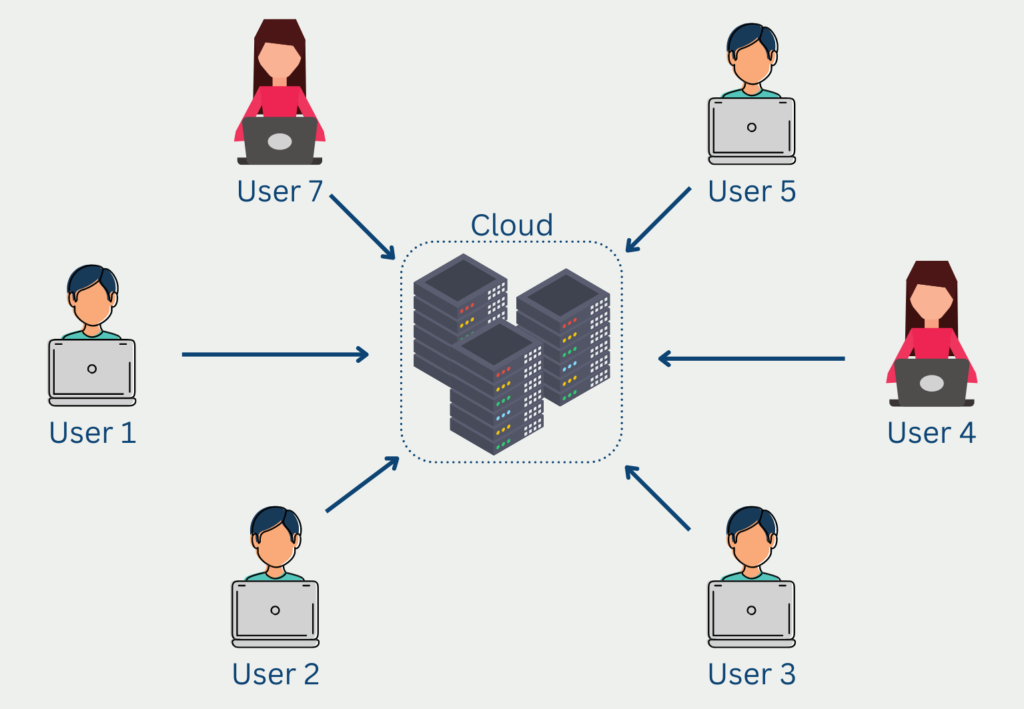
Another important benefit of RESTful APIs is that they are highly scalable. Because they rely on standard HTTP methods and data formats, it is relatively easy to build applications that can handle a large number of requests and scale up or down as needed.
Finally, RESTful APIs are highly flexible. Because they rely on a few basic HTTP methods, they can be used to build a wide range of different types of applications, from mobile apps to web applications to IoT devices.
How to design such an interface?
Designing a RESTful API requires careful planning and consideration of a variety of factors. Here are some steps to follow:
- Define the resources: Determine the key resources that the API will expose. Resources can be anything that can be identified by a unique URL, such as users, products, or orders.
- Define the endpoints: For each resource, define the endpoints that will be exposed by the API. These endpoints should be based on standard HTTP methods, such as GET, POST, PUT, and DELETE.
- Choose the response format: Determine the format in which the API will return data. Common formats include JSON, XML, and HTML.
- Use consistent URLs: Use consistent URLs for resources and endpoints throughout the API. This makes the API more intuitive and easier to use.
- Use standard HTTP methods: Use standard HTTP methods for each endpoint. For example, use GET for retrieving data, POST for creating new data, PUT for updating existing data, and DELETE for deleting data.
- Use HTTP status codes: Use HTTP status codes to indicate the success or failure of API requests. For example, use 200 for successful requests, 400 for bad requests, and 404 for requests that can’t be found.
- Use authentication and authorization: Use authentication and authorization mechanisms to ensure that only authorized users can access sensitive data or perform certain actions.
- Provide documentation: Provide detailed documentation for the API, including information about resources, endpoints, response formats, and authentication/authorization mechanisms.
By following these steps, you can design a RESTful API that is easy to use, consistent, and secure.
Which tools can be used for the implementation?
There are several technologies and tools that can be used to implement a RESTful API, each with its own set of advantages and disadvantages. Here are some popular options:
- Flask: Flask is a lightweight Python web framework that is commonly used to build RESTful APIs. It provides a simple and flexible way to create endpoints and supports a variety of extensions for authentication, database integration, and more.
- Node.js: Node.js is a popular JavaScript runtime that is often used to build scalable and performant RESTful APIs. It has a large ecosystem of packages and frameworks, such as Express.js, that make it easy to build and deploy APIs quickly.
- Django: Django is a full-stack Python web framework that includes built-in support for creating RESTful APIs through its Django REST framework. It provides a powerful set of tools for serialization, authentication, and more.
- Ruby on Rails: Ruby on Rails is a popular web framework that is often used to build RESTful APIs. It has a strong focus on convention over configuration, making it easy to get up and running quickly. It also includes built-in support for database integration and other common tasks.
- ASP.NET: ASP.NET is a Microsoft framework for building web applications, including RESTful APIs. It includes a variety of features and tools for authentication, caching, and other tasks.
Ultimately, the choice of technology or tool will depend on a variety of factors, such as the specific requirements of the project, the expertise of the development team, and the scalability and performance needs of the API.
How to secure the API?
When it comes to security considerations for RESTful APIs, there are several important factors to keep in mind. Firstly, it’s crucial to implement proper authentication and authorization mechanisms to ensure that only authorized users can access the API’s resources. This can be achieved through the use of techniques like API keys, OAuth, or token-based authentication.
Another important consideration is to implement proper data validation and sanitization to prevent common security vulnerabilities like injection attacks. This can be done through the use of validation libraries or frameworks, as well as input sanitization techniques like parameterized queries.
Additionally, it’s important to consider the potential for attacks like CSRF (Cross-Site Request Forgery) and XSS (Cross-Site Scripting). Measures like using CSRF tokens and sanitizing user input can help mitigate these risks.
Other security considerations include the use of SSL/TLS encryption to protect data in transit, as well as implementing rate limiting and other measures to prevent abuse and DDoS attacks.
Overall, implementing robust security measures is essential for ensuring that RESTful APIs are secure and can be used safely by authorized users.
What are the differences between RESTful API and OpenAPI?
RESTful API (Representational State Transfer API) is an architectural style used to build web services. It defines a set of constraints and principles for creating web services that are scalable, efficient, and easy to maintain. RESTful APIs use HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations on resources exposed by the API.
OpenAPI, on the other hand, is a specification for building APIs. It defines a standard way of describing RESTful APIs, including the endpoints, request/response formats, parameters, and other details. OpenAPI is based on JSON or YAML and is used to build documentation and client SDKs for APIs.
In essence, RESTful API is a design pattern for building APIs, while OpenAPI is a specification for documenting and describing APIs built using the RESTful pattern.
How can you call REST APIs in Python?
In modern software development, interacting with RESTful APIs (Representational State Transfer) has become a fundamental aspect. RESTful APIs enable communication between different systems over the internet by using standard HTTP methods (GET, POST, PUT, DELETE) and exchanging data in formats like JSON or XML. Python provides several libraries and frameworks to simplify the process of consuming RESTful APIs.
Using the requests Library:
One of the most popular libraries for making HTTP requests in Python is the requests
library. It provides a simple and elegant API for interacting with web services. Below is a basic example of how to make GET and POST requests using the requests
library:
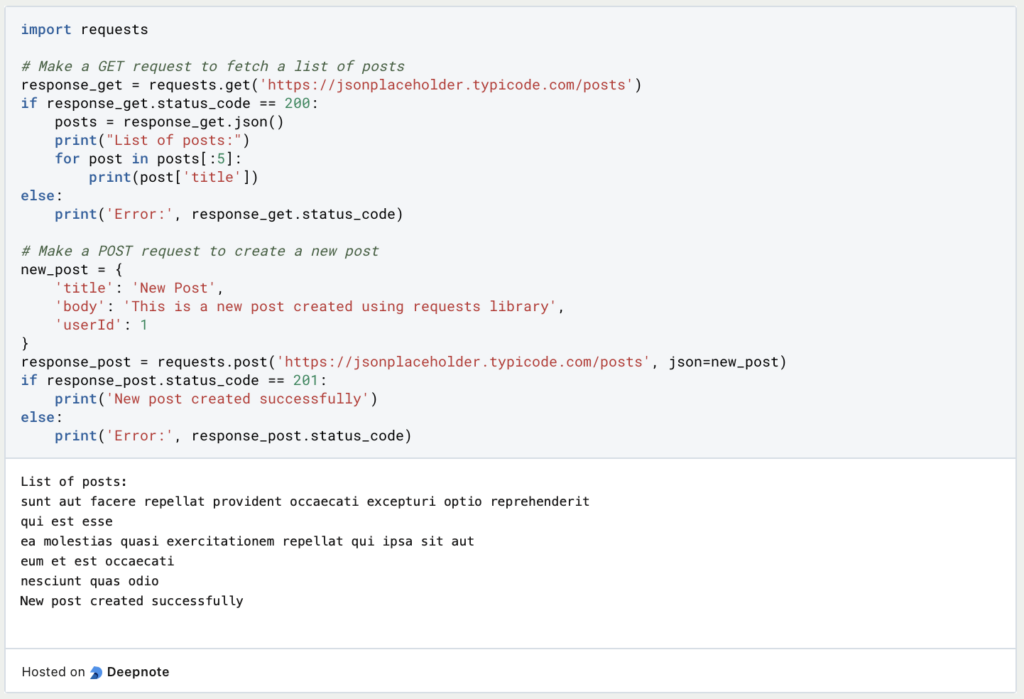
Using the urllib Library:
Python’s built-in urllib
module can also be used to make HTTP requests, although it is generally considered less user-friendly than requests
. Here’s an example of making a GET request with urllib
:
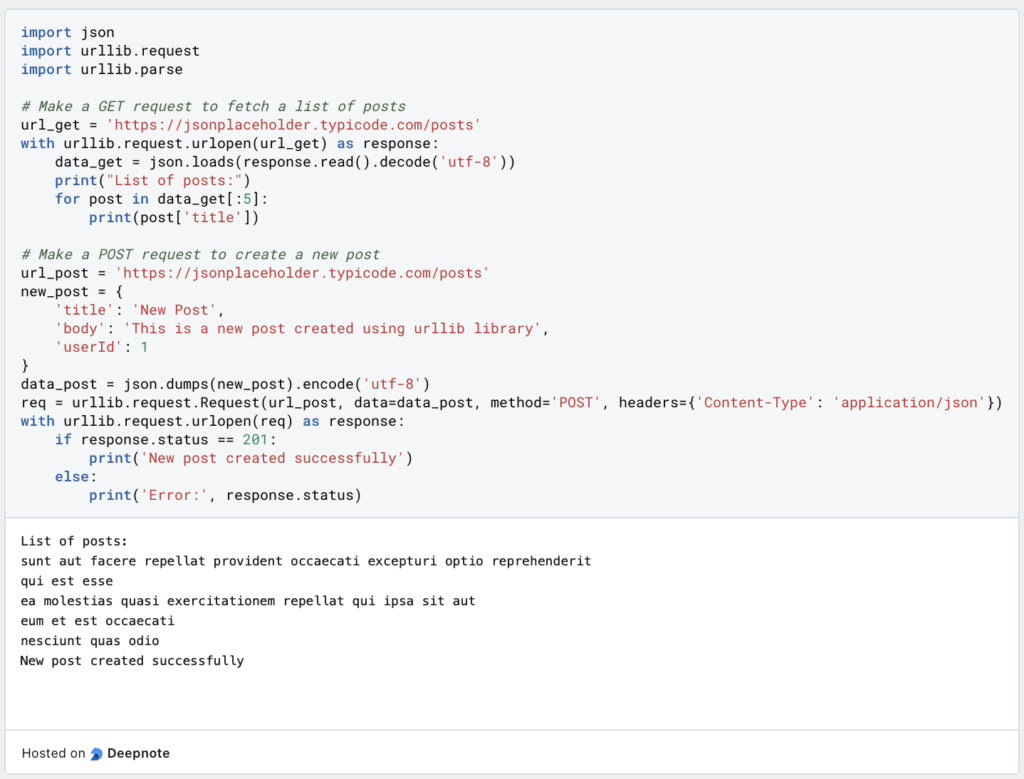
In this section, we’ve explored different ways to call RESTful APIs in Python using various libraries. Depending on your specific requirements and preferences, you can choose the approach that best fits your needs. The requests
library is recommended for most cases due to its simplicity and ease of use, but the other methods provide alternatives for different use cases or scenarios where external dependencies are not desirable.
This is what you should take with you
- RESTful APIs provide a standardized approach to web service design, making it easier to develop and maintain web applications.
- Designing such an interface involves carefully defining resources, endpoints, and methods, as well as considering factors such as security and scalability.
- Security considerations are crucial when developing these APIs, including authentication and authorization.
- Real-world applications are diverse, including e-commerce, social media, and healthcare.
- While RESTful APIs and OpenAPI are related, they serve different purposes, with OpenAPI focusing on API documentation and specification.
Thanks to Deepnote for sponsoring this article! Deepnote offers me the possibility to embed Python code easily and quickly on this website and also to host the related notebooks in the cloud.
What is the Univariate Analysis?
Master Univariate Analysis: Dive Deep into Data with Visualization, and Python - Learn from In-Depth Examples and Hands-On Code.
What is OpenAPI?
Explore OpenAPI: A Comprehensive Guide to Building and Consuming RESTful APIs. Learn How to Design, Document, and Test APIs.
What is Data Governance?
Ensure the quality, availability, and integrity of your organization's data through effective data governance. Learn more here.
What is Data Quality?
Ensuring Data Quality: Importance, Challenges, and Best Practices. Learn how to maintain high-quality data to drive better business decisions.
What is Data Imputation?
Impute missing values with data imputation techniques. Optimize data quality and learn more about the techniques and importance.
What is Outlier Detection?
Discover hidden anomalies in your data with advanced outlier detection techniques. Improve decision-making and uncover valuable insights.
Other Articles on the Topic of Restful APIs
This link will get you to my Deepnote App where you can find all the code that I used in this article and can run it yourself.

Niklas Lang
I have been working as a machine learning engineer and software developer since 2020 and am passionate about the world of data, algorithms and software development. In addition to my work in the field, I teach at several German universities, including the IU International University of Applied Sciences and the Baden-Württemberg Cooperative State University, in the fields of data science, mathematics and business analytics.
My goal is to present complex topics such as statistics and machine learning in a way that makes them not only understandable, but also exciting and tangible. I combine practical experience from industry with sound theoretical foundations to prepare my students in the best possible way for the challenges of the data world.